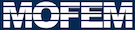 |
| v0.14.0
|
- Examples
- plate.cpp.
Definition at line 141 of file plate.cpp.
◆ Plate()
◆ assembleSystem()
[Boundary condition]
[Push operators to pipeline]
calculate jacobian
- Examples
- plate.cpp.
Definition at line 235 of file plate.cpp.
240 auto rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * p; };
241 auto rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * p; };
244 CHKERR pipeline_mng->setDomainLhsIntegrationRule(rule_lhs);
245 CHKERR pipeline_mng->setDomainRhsIntegrationRule(rule_rhs);
247 CHKERR pipeline_mng->setSkeletonLhsIntegrationRule(rule_2);
248 CHKERR pipeline_mng->setSkeletonRhsIntegrationRule(rule_2);
249 CHKERR pipeline_mng->setBoundaryLhsIntegrationRule(rule_2);
250 CHKERR pipeline_mng->setBoundaryRhsIntegrationRule(rule_2);
252 auto det_ptr = boost::make_shared<VectorDouble>();
253 auto jac_ptr = boost::make_shared<MatrixDouble>();
254 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
255 auto base_mass_ptr = boost::make_shared<MatrixDouble>();
256 auto data_l2_ptr = boost::make_shared<EntitiesFieldData>(MBENTITYSET);
260 auto push_ho_direcatives = [&](
auto &pipeline) {
264 BaseDerivatives::SecondDerivative, base_mass_ptr, data_l2_ptr,
272 auto push_jacobian = [&](
auto &pipeline) {
283 push_ho_direcatives(pipeline_mng->getOpDomainLhsPipeline());
284 push_jacobian(pipeline_mng->getOpDomainLhsPipeline());
286 pipeline_mng->getOpDomainLhsPipeline().push_back(
291 pipeline_mng->getOpDomainRhsPipeline().push_back(
295 auto side_fe_ptr = boost::make_shared<FaceSideEle>(
mField);
296 push_ho_direcatives(side_fe_ptr->getOpPtrVector());
297 push_jacobian(side_fe_ptr->getOpPtrVector());
301 pipeline_mng->getOpSkeletonLhsPipeline().push_back(
◆ boundaryCondition()
[Set up problem]
[Boundary condition]
- Examples
- plate.cpp.
Definition at line 200 of file plate.cpp.
204 auto get_skin = [&]() {
210 MOAB_THROW(skin.find_skin(0, body_ents,
false, skin_ents));
213 skin_ents.merge(verts);
218 auto remove_dofs_from_problem = [&](
Range &&skin) {
222 CHKERR problem_mng->removeDofsOnEntities(
simple->getProblemName(),
"U",
228 CHKERR remove_dofs_from_problem(get_skin());
◆ outputResults()
[Solve system]
[Output results]
- Examples
- plate.cpp.
Definition at line 336 of file plate.cpp.
341 pipeline_mng->getSkeletonRhsFE().reset();
342 pipeline_mng->getSkeletonLhsFE().reset();
343 pipeline_mng->getBoundaryRhsFE().reset();
344 pipeline_mng->getBoundaryLhsFE().reset();
346 auto post_proc_fe = boost::make_shared<PostProcEle>(
mField);
348 auto u_ptr = boost::make_shared<VectorDouble>();
349 post_proc_fe->getOpPtrVector().push_back(
354 post_proc_fe->getOpPtrVector().push_back(
356 new OpPPMap(post_proc_fe->getPostProcMesh(),
357 post_proc_fe->getMapGaussPts(),
367 pipeline_mng->getDomainRhsFE() = post_proc_fe;
368 CHKERR pipeline_mng->loopFiniteElements();
369 CHKERR post_proc_fe->writeFile(
"out_result.h5m");
◆ readMesh()
◆ runProblem()
◆ setupProblem()
◆ solveSystem()
[Push operators to pipeline]
[Solve system]
- Examples
- plate.cpp.
Definition at line 309 of file plate.cpp.
315 auto ksp_solver = pipeline_mng->createKSP();
316 CHKERR KSPSetFromOptions(ksp_solver);
317 CHKERR KSPSetUp(ksp_solver);
320 auto dm =
simple->getDM();
327 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
328 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
◆ mField
The documentation for this struct was generated from the following file:
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
Operator tp collect data from elements on the side of Edge/Face.
auto plate_stiffness
get fourth-order constitutive tensor
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< BASE_DIM, FIELD_DIM > OpDomainPlateLoad
Problem manager is used to build and partition problems.
constexpr int SPACE_DIM
dimension of space
constexpr int FIELD_DIM
dimension of approx. field
@ L2
field with C-1 continuity
PipelineManager interface.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
#define MOAB_THROW(err)
Check error code of MoAB function and throw MoFEM exception.
MoFEMErrorCode readMesh()
[Read mesh]
Simple interface for fast problem set-up.
#define CHKERR
Inline error check.
auto createDMVector(DM dm)
Get smart vector from DM.
virtual moab::Interface & get_moab()=0
void simple(double P1[], double P2[], double P3[], double c[], const int N)
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
MoFEM::Interface & mField
Get value at integration points for scalar field.
Modify integration weights on face to take in account higher-order geometry.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpGradGradSymTensorGradGrad< 1, 1, SPACE_DIM, 0 > OpDomainPlateStiffness
MoFEMErrorCode setupProblem()
[Read mesh]
boost::shared_ptr< FEMethod > & getDomainLhsFE()
#define MOFEM_LOG(channel, severity)
Log.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
MoFEMErrorCode solveSystem()
[Push operators to pipeline]
MoFEMErrorCode assembleSystem()
[Boundary condition]
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const double D
diffusivity
PetscErrorCode PetscOptionsGetScalar(PetscOptions *, const char pre[], const char name[], PetscScalar *dval, PetscBool *set)
MoFEMErrorCode boundaryCondition()
[Set up problem]
Operator the left hand side matrix.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode outputResults()
[Solve system]
Post post-proc data at points from hash maps.