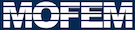 |
| v0.14.0
|
Go to the documentation of this file.
13 using namespace boost::numeric;
14 using namespace MoFEM;
18 int main(
int argc,
char *argv[]) {
32 PetscBool ale = PETSC_FALSE;
34 PetscBool test_jacobian = PETSC_FALSE;
48 if (ale == PETSC_TRUE) {
67 if (ale == PETSC_TRUE) {
73 boost::shared_ptr<ForcesAndSourcesCore> fe_lhs_ptr(
75 boost::shared_ptr<ForcesAndSourcesCore> fe_rhs_ptr(
78 int operator()(
int,
int,
int)
const {
return 2 * (
order - 1); }
80 fe_lhs_ptr->getRuleHook =
VolRule();
81 fe_rhs_ptr->getRuleHook =
VolRule();
89 boost::shared_ptr<map<int, BlockData>> block_sets_ptr =
90 boost::make_shared<map<int, BlockData>>();
91 (*block_sets_ptr)[0].
iD = 0;
92 (*block_sets_ptr)[0].E = 1;
93 (*block_sets_ptr)[0].PoissonRatio = 0.25;
98 "x",
"X", ale,
false);
101 CHKERR DMCreateGlobalVector(dm, &x);
114 CHKERR MatDuplicate(
A, MAT_DO_NOT_COPY_VALUES, &fdA);
116 if (test_jacobian == PETSC_TRUE) {
117 char testing_options[] =
118 "-snes_test_jacobian -snes_test_jacobian_display "
119 "-snes_no_convergence_test -snes_atol 0 -snes_rtol 0 -snes_max_it 1 "
121 CHKERR PetscOptionsInsertString(NULL, testing_options);
123 char testing_options[] =
"-snes_no_convergence_test -snes_atol 0 "
124 "-snes_rtol 0 -snes_max_it 1 -pc_type none";
125 CHKERR PetscOptionsInsertString(NULL, testing_options);
129 CHKERR SNESCreate(PETSC_COMM_WORLD, &snes);
134 CHKERR SNESSetFromOptions(snes);
136 CHKERR SNESSolve(snes, NULL, x);
138 if (test_jacobian == PETSC_FALSE) {
140 CHKERR MatNorm(
A, NORM_INFINITY, &nrm_A0);
142 char testing_options_fd[] =
"-snes_fd";
143 CHKERR PetscOptionsInsertString(NULL, testing_options_fd);
147 CHKERR SNESSetFromOptions(snes);
149 CHKERR SNESSolve(snes, NULL, x);
150 CHKERR MatAXPY(
A, -1, fdA, SUBSET_NONZERO_PATTERN);
153 CHKERR MatNorm(
A, NORM_INFINITY, &nrm_A);
154 PetscPrintf(PETSC_COMM_WORLD,
"Matrix norms %3.4e %3.4e\n", nrm_A,
158 const double tol = 1e-5;
161 "Difference between hand-calculated tangent matrix and finite "
162 "difference matrix is too big");
170 CHKERR SNESDestroy(&snes);
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
PetscErrorCode DMMoFEMGetSnesCtx(DM dm, MoFEM::SnesCtx **snes_ctx)
get MoFEM::SnesCtx data structure
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
const std::string getDomainFEName() const
Get the Domain FE Name.
int main(int argc, char *argv[])
PetscErrorCode DMMoFEMSNESSetJacobian(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES Jacobian evaluation function
Volume finite element base.
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
virtual MoFEMErrorCode get_finite_element_entities_by_dimension(const std::string name, int dim, Range &ents) const =0
get entities in the finite element by dimension
PetscErrorCode SnesRhs(SNES snes, Vec x, Vec f, void *ctx)
This is MoFEM implementation for the right hand side (residual vector) evaluation in SNES solver.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const FTensor::Tensor2< T, Dim, Dim > Vec
static MoFEMErrorCode setOperators(boost::shared_ptr< ForcesAndSourcesCore > fe_lhs_ptr, boost::shared_ptr< ForcesAndSourcesCore > fe_rhs_ptr, boost::shared_ptr< map< int, BlockData >> block_sets_ptr, const std::string x_field, const std::string X_field, const bool ale, const bool field_disp, const EntityType type=MBTET, boost::shared_ptr< DataAtIntegrationPts > data_at_pts=nullptr)
@ MOFEM_ATOM_TEST_INVALID
Interface for nonlinear (SNES) solver.
PetscErrorCode SnesMat(SNES snes, Vec x, Mat A, Mat B, void *ctx)
This is MoFEM implementation for the left hand side (tangent matrix) evaluation in SNES solver.
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
NonlinearElasticElement::BlockData BlockData
PetscErrorCode DMMoFEMSNESSetFunction(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES residual evaluation function
PetscErrorCode PetscOptionsGetBool(PetscOptions *, const char pre[], const char name[], PetscBool *bval, PetscBool *set)