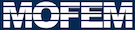 |
| v0.14.0
|
Go to the documentation of this file.
20 static char help[] =
"...\n\n";
21 static const bool debug =
false;
36 double operator()(
const double x,
const double y,
const double z)
const {
37 return 1 + x * x + y * y + z * z * z;
46 const double z)
const {
68 double operator()(
const double x,
const double y,
const double z)
const {
75 OpS(
const bool beta = 1)
108 if (row_side == col_side && row_type == col_type) {
155 const double alpha = t_w * area;
160 for (
int rr = 0; rr !=
nbRows; rr++) {
164 for (
int cc = 0; cc !=
nbCols; cc++) {
166 a += alpha * t_row_base * t_col_base;
188 const int *row_indices = &*row_data.
getIndices().data().begin();
190 const int *col_indices = &*col_data.
getIndices().data().begin();
195 &*
locMat.data().begin(), ADD_VALUES);
201 &*
locMat.data().begin(), ADD_VALUES);
207 int main(
int argc,
char *argv[]) {
219 PetscBool flg_test = PETSC_FALSE;
220 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"",
"Poisson's problem options",
226 CHKERR PetscOptionsBool(
"-test",
"if true is ctest",
"", flg_test,
227 &flg_test, PETSC_NULL);
228 ierr = PetscOptionsEnd();
248 boost::shared_ptr<ForcesAndSourcesCore>
250 boost::shared_ptr<ForcesAndSourcesCore>
252 boost::shared_ptr<ForcesAndSourcesCore>
254 boost::shared_ptr<ForcesAndSourcesCore>
256 boost::shared_ptr<ForcesAndSourcesCore>
258 boost::shared_ptr<PoissonExample::PostProcFE>
260 boost::shared_ptr<ForcesAndSourcesCore>
null;
261 boost::shared_ptr<ForcesAndSourcesCore> boundary_penalty_lhs_fe;
268 boundary_lhs_fe, domain_rhs_fe, boundary_rhs_fe,
false);
273 global_error, domain_error);
278 const double beta = 1;
279 boundary_penalty_lhs_fe = boost::shared_ptr<ForcesAndSourcesCore>(
282 boundary_penalty_lhs_fe->getOpPtrVector().push_back(
new OpS(beta));
283 boundary_rhs_fe->getOpPtrVector().push_back(
358 CHKERR DMCreateGlobalVector(dm, &
F);
363 DM dm_sub_KK, dm_sub_LU;
364 ublas::matrix<Mat> nested_matrices(2, 2);
365 ublas::vector<IS> nested_is(2);
367 CHKERR DMCreate(PETSC_COMM_WORLD, &dm_sub_KK);
368 CHKERR DMSetType(dm_sub_KK,
"DMMOFEM");
370 CHKERR DMSetFromOptions(dm_sub_KK);
378 CHKERR DMSetUp(dm_sub_KK);
380 CHKERR DMCreateMatrix(dm_sub_KK, &nested_matrices(0, 0));
381 domain_lhs_fe->ksp_B = nested_matrices(0, 0);
384 boundary_penalty_lhs_fe->ksp_B = nested_matrices(0, 0);
387 boundary_penalty_lhs_fe);
388 CHKERR MatAssemblyBegin(nested_matrices(0, 0), MAT_FINAL_ASSEMBLY);
389 CHKERR MatAssemblyEnd(nested_matrices(0, 0), MAT_FINAL_ASSEMBLY);
390 CHKERR DMDestroy(&dm_sub_KK);
392 CHKERR DMCreate(PETSC_COMM_WORLD, &dm_sub_LU);
393 CHKERR DMSetType(dm_sub_LU,
"DMMOFEM");
395 CHKERR DMSetFromOptions(dm_sub_LU);
401 CHKERR DMSetUp(dm_sub_LU);
403 CHKERR DMCreateMatrix(dm_sub_LU, &nested_matrices(1, 0));
404 boundary_lhs_fe->ksp_B = nested_matrices(1, 0);
407 CHKERR MatAssemblyBegin(nested_matrices(1, 0), MAT_FINAL_ASSEMBLY);
408 CHKERR MatAssemblyEnd(nested_matrices(1, 0), MAT_FINAL_ASSEMBLY);
410 CHKERR MatTranspose(nested_matrices(1, 0), MAT_INITIAL_MATRIX,
411 &nested_matrices(0, 1));
412 CHKERR DMDestroy(&dm_sub_LU);
414 domain_rhs_fe->ksp_f =
F;
417 boundary_rhs_fe->ksp_f =
F;
424 nested_matrices(1, 1) = PETSC_NULL;
428 MatGetType(nested_matrices(0, 0), &
type);
429 cerr <<
"K " <<
type << endl;
430 MatGetType(nested_matrices(1, 0), &
type);
431 cerr <<
"C " <<
type << endl;
432 MatGetType(nested_matrices(0, 1), &
type);
433 cerr <<
"CT " <<
type << endl;
435 cerr <<
"UU" << endl;
436 MatView(nested_matrices(0, 0), PETSC_VIEWER_DRAW_WORLD);
438 cerr <<
"LU" << endl;
439 MatView(nested_matrices(1, 0), PETSC_VIEWER_DRAW_WORLD);
441 cerr <<
"UL" << endl;
442 MatView(nested_matrices(0, 1), PETSC_VIEWER_DRAW_WORLD);
446 CHKERR MatCreateNest(PETSC_COMM_WORLD, 2, &nested_is[0], 2, &nested_is[0],
447 &nested_matrices(0, 0), &
A);
451 CHKERR KSPCreate(PETSC_COMM_WORLD, &solver);
452 CHKERR KSPSetFromOptions(solver);
454 CHKERR KSPSetOperators(solver,
A,
A);
456 CHKERR KSPGetPC(solver, &pc);
457 PetscBool is_pcfs = PETSC_FALSE;
458 PetscObjectTypeCompare((PetscObject)pc, PCFIELDSPLIT, &is_pcfs);
460 CHKERR PCFieldSplitSetIS(pc, NULL, nested_is[0]);
461 CHKERR PCFieldSplitSetIS(pc, NULL, nested_is[1]);
464 "Only works with pre-conditioner PCFIELDSPLIT");
478 CHKERR KSPDestroy(&solver);
479 for (
int i = 0;
i != 2;
i++) {
480 CHKERR ISDestroy(&nested_is[
i]);
481 for (
int j = 0;
j != 2;
j++) {
482 CHKERR MatDestroy(&nested_matrices(
i,
j));
499 if (flg_test == PETSC_TRUE) {
510 post_proc_volume->writeFile(
"out_vol.h5m");
517 CHKERR VecDestroy(&global_error);
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Data on single entity (This is passed as argument to DataOperator::doWork)
MoFEMErrorCode MatSetValues(Mat M, const EntitiesFieldData::EntData &row_data, const EntitiesFieldData::EntData &col_data, const double *ptr, InsertMode iora)
Assemble PETSc matrix.
PetscErrorCode DMMoFEMAddSubFieldCol(DM dm, const char field_name[])
PetscErrorCode DMMoFEMSetSquareProblem(DM dm, PetscBool square_problem)
set squared problem
@ L2
field with C-1 continuity
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
UBlasMatrix< double > MatrixDouble
Mat & snes_B
preconditioner of jacobian matrix
FTensor::Index< 'i', 3 > i
summit Index
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
MoFEMErrorCode iNtegrate(EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
Integrate grad-grad operator.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Create finite elements instances.
Simple interface for fast problem set-up.
@ OPROWCOL
operator doWork is executed on FE rows &columns
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
auto getFTensor0IntegrationWeight()
Get integration weights.
Deprecated interface functions.
DeprecatedCoreInterface Interface
MatrixDouble & getGaussPts()
matrix of integration (Gauss) points for Volume Element
FTensor::Tensor0< FTensor::PackPtr< double *, 1 > > getFTensor0N(const FieldApproximationBase base)
Get base function as Tensor0.
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode createGhostVec(Vec *ghost_vec) const
double operator()(const double x, const double y, const double z) const
double operator()(const double x, const double y, const double z) const
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
Assemble constrains vector.
MoFEMErrorCode creatFEToPostProcessResults(boost::shared_ptr< PostProcFE > &post_proc_volume) const
Create finite element to post-process results.
int nbRows
number of dofs on rows
bool isDiag
true if this block is on diagonal
default operator for TRI element
const double bEta
error code
PetscErrorCode DMMoFEMCreateSubDM(DM subdm, DM dm, const char problem_name[])
Must be called by user to set Sub DM MoFEM data structures.
PetscErrorCode DMMoFEMGetSubRowIS(DM dm, IS *is)
get sub problem is
MoFEMErrorCode testError(Vec ghost_vec)
Test error.
PetscErrorCode DMoFEMMeshToGlobalVector(DM dm, Vec g, InsertMode mode, ScatterMode scatter_mode)
set ghosted vector values on all existing mesh entities
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
const VectorInt & getIndices() const
Get global indices of dofs on entity.
MatrixDouble locMat
local entity block matrix
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
const std::string getDomainFEName() const
Get the Domain FE Name.
int nbCols
number if dof on column
MoFEMErrorCode printError(Vec ghost_vec)
Print error.
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
Do calculations for give operator.
FTensor::Index< 'i', SPACE_DIM > i
MoFEMErrorCode addDataField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
const FEMethod * getFEMethod() const
Return raw pointer to Finite Element Method object.
int nbIntegrationPts
number of integration points
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
FTensor::Index< 'j', 3 > j
MoFEMErrorCode createFEToEvaluateError(boost::function< double(const double, const double, const double)> f_u, boost::function< FTensor::Tensor1< double, 3 >(const double, const double, const double)> g_u, Vec global_error, boost::shared_ptr< ForcesAndSourcesCore > &domain_error) const
Create finite element to calculate error.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const std::string getBoundaryFEName() const
Get the Boundary FE Name.
const FTensor::Tensor2< T, Dim, Dim > Vec
@ MOFEM_DATA_INCONSISTENCY
Set integration rule to boundary elements.
int main(int argc, char *argv[])
MoFEMErrorCode addBoundaryField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on boundary.
const double D
diffusivity
MoFEMErrorCode createFEToAssembleMatrixAndVector(boost::function< double(const double, const double, const double)> f_u, boost::function< double(const double, const double, const double)> f_source, boost::shared_ptr< ForcesAndSourcesCore > &domain_lhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &boundary_lhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &domain_rhs_fe, boost::shared_ptr< ForcesAndSourcesCore > &boundary_rhs_fe, bool trans=true) const
Create finite element to calculate matrix and vectors.
PetscErrorCode DMMoFEMAddSubFieldRow(DM dm, const char field_name[])
double getArea()
get area of face
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode assembleGhostVector(Vec ghost_vec) const
Assemble error vector.
FTensor::Tensor1< double, 3 > operator()(const double x, const double y, const double z) const
MoFEMErrorCode aSsemble(EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
Assemble local entity block matrix.