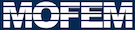 |
| v0.14.0
|
Go to the documentation of this file.
18 using namespace MoFEM;
26 #include <boost/tokenizer.hpp>
30 using namespace boost;
35 int main(
int argc,
char *argv[]) {
45 char data_file_name1[255];
46 char data_file_name2[255];
47 PetscBool flg_file = PETSC_FALSE;
49 PetscBool flg_n_part = PETSC_FALSE;
50 PetscInt n_partas = 1;
52 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"",
"none",
"none");
53 CHKERR PetscOptionsString(
"-my_file",
"mesh file name",
"",
"mesh.h5m",
56 CHKERR PetscOptionsString(
"-my_data_x",
"data file name",
"",
"data_x.h5m",
57 data_file_name1, 255, PETSC_NULL);
59 CHKERR PetscOptionsString(
"-my_data_y",
"data file name",
"",
"data_y.h5m",
60 data_file_name2, 255, PETSC_NULL);
62 CHKERR PetscOptionsInt(
"-my_order",
"default approximation order",
"", 1,
65 CHKERR PetscOptionsInt(
"-my_nparts",
"number of parts",
"", 1, &n_partas,
68 ierr = PetscOptionsEnd();
71 if (flg_file != PETSC_TRUE) {
72 SETERRQ(PETSC_COMM_SELF, 1,
"*** ERROR -my_file (MESH FILE NEEDED)");
75 if (flg_n_part != PETSC_TRUE) {
76 SETERRQ(PETSC_COMM_SELF, 1,
"*** ERROR partitioning number not given");
82 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
84 pcomm =
new ParallelComm(&moab, PETSC_COMM_WORLD);
141 DMType dm_name =
"DM_DISP";
144 CHKERR DMCreate(PETSC_COMM_WORLD, &dm_disp);
145 CHKERR DMSetType(dm_disp, dm_name);
150 CHKERR DMSetFromOptions(dm_disp);
161 string file1(data_file_name1);
166 string file2(data_file_name2);
171 boost::ptr_vector<MoFEM::ForcesAndSourcesCore::UserDataOperator>
176 CHKERR DMCreateMatrix(dm_disp, &
A);
182 CHKERR VecDuplicate(Fx, &Fy);
183 CHKERR VecDuplicate(Dx, &Dy);
186 CHKERR VecZeroEntries(Fx);
187 CHKERR VecZeroEntries(Fy);
192 common_data.
globF = Fx;
193 list_of_operators.push_back(
194 new OpCalculateInvJacForFace(common_data.
invJac));
196 list_of_operators.push_back(
197 new OpCalculateLhs(
"DISP_X",
"DISP_X", common_data, data_from_files));
198 list_of_operators.push_back(
200 list_of_operators.push_back(
new OpAssmbleRhs(
"DISP_X", common_data));
205 CHKERR MatAssemblyBegin(
A, MAT_FINAL_ASSEMBLY);
206 CHKERR MatAssemblyEnd(
A, MAT_FINAL_ASSEMBLY);
207 CHKERR VecGhostUpdateBegin(Fx, ADD_VALUES, SCATTER_REVERSE);
208 CHKERR VecGhostUpdateEnd(Fx, ADD_VALUES, SCATTER_REVERSE);
209 CHKERR VecAssemblyBegin(Fx);
210 CHKERR VecAssemblyEnd(Fx);
213 common_data.
globF = Fy;
214 list_of_operators.clear();
215 list_of_operators.push_back(
216 new OpCalculateInvJacForFace(common_data.
invJac));
218 list_of_operators.push_back(
220 list_of_operators.push_back(
new OpAssmbleRhs(
"DISP_X", common_data));
225 CHKERR VecGhostUpdateBegin(Fy, ADD_VALUES, SCATTER_REVERSE);
226 CHKERR VecGhostUpdateEnd(Fy, ADD_VALUES, SCATTER_REVERSE);
227 CHKERR VecAssemblyBegin(Fy);
228 CHKERR VecAssemblyEnd(Fy);
238 CHKERR KSPCreate(PETSC_COMM_WORLD, &ksp);
240 CHKERR KSPSetFromOptions(ksp);
241 CHKERR KSPSolve(ksp, Fx, Dx);
242 CHKERR KSPSolve(ksp, Fy, Dy);
245 CHKERR VecGhostUpdateBegin(Dx, INSERT_VALUES, SCATTER_FORWARD);
246 CHKERR VecGhostUpdateEnd(Dx, INSERT_VALUES, SCATTER_FORWARD);
248 "DM_DISP",
COL, Dx, INSERT_VALUES, SCATTER_REVERSE);
249 CHKERR VecGhostUpdateBegin(Dy, INSERT_VALUES, SCATTER_FORWARD);
250 CHKERR VecGhostUpdateEnd(Dy, INSERT_VALUES, SCATTER_FORWARD);
252 "DM_DISP",
"DISP_X",
"DISP_Y",
COL, Dy, INSERT_VALUES, SCATTER_REVERSE);
259 sum_dx += (*dof)->getFieldData();
265 (*dof)->getFieldData() -= sum_dx;
271 sum_dy += (*dof)->getFieldData();
277 (*dof)->getFieldData() -= sum_dy;
287 "PARALLEL=WRITE_PART");
294 CHKERR DMDestroy(&dm_disp);
303 Range ents_1st_layer;
305 ents_1st_layer,
true);
308 CHKERR moab.get_connectivity(ents_1st_layer[0], conn, num_nodes);
311 CHKERR skin.find_skin(0, ents_1st_layer,
false, skin_edges);
313 CHKERR moab.get_adjacencies(&*ents_1st_layer.begin(), 1, 1,
false, edges);
316 CHKERR moab.add_entities(meshset, conn, 1);
317 CHKERR moab.add_entities(meshset, skin_edges);
322 CHKERR moab.get_entities_by_type(0, MBTET, tets,
false);
326 CHKERR moab.write_file(
"analysis_mesh.h5m");
#define MYPCOMM_INDEX
default communicator number PCOMM
moab::Interface & postProcMesh
virtual MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const =0
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
MoFEMErrorCode fromOptions()
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
Deprecated interface functions.
int main(int argc, char *argv[])
MoFEMErrorCode getMeshset(const int ms_id, const unsigned int cubit_bc_type, EntityHandle &meshset) const
get meshset from CUBIT Id and CUBIT type
DeprecatedCoreInterface Interface
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string &name, const bool recursive=true)=0
add entities to finite element
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
MoFEMErrorCode getEntitiesByDimension(const int ms_id, const unsigned int cubit_bc_type, const int dimension, Range &entities, const bool recursive=true) const
get entities from CUBIT/meshset of a particular entity dimension
virtual MoFEMErrorCode add_field(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
def surface(x, y, z, eta)
virtual MoFEMErrorCode delete_finite_element(const std::string name, int verb=DEFAULT_VERBOSITY)=0
delete finite element from mofem database
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
Post-process fields on refined mesh.
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
Vector manager is used to create vectors \mofem_vectors.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
boost::ptr_deque< UserDataOperator > & getOpPtrVector()
Use to push back operator for row operator.
const FTensor::Tensor2< T, Dim, Dim > Vec
MoFEMErrorCode loadFileData()
Interface for managing meshsets containing materials and boundary conditions.
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
DEPRECATED MoFEMErrorCode seed_ref_level_3D(const EntityHandle meshset, const BitRefLevel &bit, int verb=-1)
seed 2D entities in the meshset and their adjacencies (only TETs adjacencies) in a particular BitRefL...
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_filed)=0
set finite element field data
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
DEPRECATED MoFEMErrorCode partition_mesh(const Range &ents, const int dim, const int adj_dim, const int n_parts, int verb=-1)
Set partition tag to each finite element in the problem.
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
#define _IT_GET_DOFS_FIELD_BY_NAME_AND_TYPE_FOR_LOOP_(MFIELD, NAME, TYPE, IT)
loop over all dofs from a moFEM field and particular field
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
MoFEMErrorCode generateReferenceElementMesh()
MoFEMErrorCode addFieldValuesPostProc(const std::string field_name, Vec v=PETSC_NULL)
Add operator to post-process L2, H1, Hdiv, Hcurl field value.