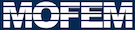 |
| v0.14.0
|
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
|
static char | help [] = "...\n\n" |
|
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
Definition at line 56 of file nonlinear_elastic_test_jacobian.cpp.
66 PetscBool flg_test_mat;
67 PetscInt choise_value =
HOOKE;
69 LASTOP, &choise_value, &flg_test_mat);
74 MPI_Comm_rank(PETSC_COMM_WORLD, &rank);
76 PetscBool flg = PETSC_TRUE;
103 if (flg != PETSC_TRUE) {
115 double scale_positions = 2;
121 if (dof_ptr->get()->getEntType() != MBVERTEX)
124 int dof_rank = dof_ptr->get()->getDofCoeffIdx();
125 double &fval = dof_ptr->get()->getFieldData();
127 CHKERR moab.get_coords(&ent, 1, coords);
130 fval = scale_positions * coords[dof_rank];
138 FunctionsToCalculatePiolaKirchhoffI<double>>(),
140 FunctionsToCalculatePiolaKirchhoffI<adouble>>());
141 elastic.commonData.spatialPositions =
"SPATIAL_POSITION";
142 elastic.commonData.meshPositions =
"MESH_NODE_POSITIONS";
144 CHKERR elastic.addElement(
"ELASTIC",
"SPATIAL_POSITION");
171 elastic.getLoopFeRhs().getOpPtrVector().push_back(
173 "SPATIAL_POSITION", elastic.commonData));
176 std::map<int, NonlinearElasticElement::BlockData>::iterator sit =
177 elastic.setOfBlocks.begin();
178 for (; sit != elastic.setOfBlocks.end(); sit++) {
179 elastic.getLoopFeRhs().getOpPtrVector().push_back(
181 "SPATIAL_POSITION", sit->second, elastic.commonData, tag0,
182 false,
false,
false));
183 elastic.getLoopFeRhs().getOpPtrVector().push_back(
185 "SPATIAL_POSITION", sit->second, elastic.commonData, tag1,
true,
186 false,
false,
false));
189 elastic.getLoopFeRhs().getOpPtrVector().push_back(
190 new OpCheck(elastic.commonData));
193 elastic.getLoopFeRhs());
197 if (flg_test_mat == PETSC_TRUE) {
198 PetscPrintf(PETSC_COMM_WORLD,
"Testing %s\n",
200 std::map<int, NonlinearElasticElement::BlockData>::iterator sit =
201 elastic.setOfBlocks.begin();
202 for (; sit != elastic.setOfBlocks.end(); sit++) {
203 switch (choise_value) {
205 sit->second.materialDoublePtr = boost::make_shared<Hooke<double>>();
206 sit->second.materialAdoublePtr = boost::make_shared<Hooke<adouble>>();
209 sit->second.materialDoublePtr =
210 boost::make_shared<NeoHookean<double>>();
211 sit->second.materialAdoublePtr =
212 boost::make_shared<NeoHookean<adouble>>();
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
Operator performs automatic differentiation.
structure grouping operators and data used for calculation of nonlinear elastic element
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
Calculate explicit derivative of free energy.
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
#define _IT_GET_DOFS_FIELD_BY_NAME_FOR_LOOP_(MFIELD, NAME, IT)
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
const char * materials_list[]
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
MoFEMErrorCode partitionProblem(const std::string name, int verb=VERBOSE)
partition problem dofs (collective)