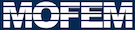 |
| v0.14.0
|
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
- Examples
- simple_elasticity.cpp.
Definition at line 390 of file simple_elasticity.cpp.
392 const string default_options =
"-ksp_type fgmres \n"
394 "-pc_factor_mat_solver_type mumps \n"
395 "-mat_mumps_icntl_20 0 \n"
398 string param_file =
"param_file.petsc";
399 if (!
static_cast<bool>(ifstream(param_file))) {
400 std::ofstream file(param_file.c_str(), std::ios::ate);
401 if (file.is_open()) {
402 file << default_options;
422 PetscBool flg_test = PETSC_FALSE;
423 CHKERR PetscOptionsBegin(PETSC_COMM_WORLD,
"",
"SimpleElasticProblem",
431 CHKERR PetscOptionsBool(
"-test",
"if true is ctest",
"", flg_test,
432 &flg_test, PETSC_NULL);
434 ierr = PetscOptionsEnd();
442 Range fix_faces, pressure_faces, fix_nodes, fix_second_node;
447 BRICK_PRESSURE_FACES = 3,
453 int id =
bit->getMeshsetId();
455 if (
id == FIX_BRICK_FACES) {
461 CHKERR m_field.
get_moab().get_adjacencies(fix_faces, 0,
false, adj_ents,
462 moab::Interface::UNION);
464 CHKERR m_field.
get_moab().get_adjacencies(fix_faces, 1,
false, adj_ents,
465 moab::Interface::UNION);
466 fix_faces.merge(adj_ents);
467 }
else if (
id == FIX_NODES) {
472 }
else if (
id == BRICK_PRESSURE_FACES) {
474 meshset, 2, pressure_faces,
true);
479 meshset, 0, fix_second_node,
true);
516 boost::shared_ptr<VolumeElementForcesAndSourcesCore> elastic_fe(
518 boost::shared_ptr<FaceElementForcesAndSourcesCore> pressure_fe(
522 elastic_fe->getRuleHook =
VolRule();
523 pressure_fe->getRuleHook =
FaceRule();
527 elastic_fe->getOpPtrVector().push_back(
new OpK());
529 pressure_fe->getOpPtrVector().push_back(
new OpPressure());
531 boost::shared_ptr<FEMethod> fix_dofs_fe(
534 boost::shared_ptr<FEMethod> null_fe;
538 dm, simple_interface->
getDomainFEName(), elastic_fe, null_fe, null_fe);
553 CHKERR DMCreateGlobalVector(dm, &x);
561 elastic_fe->ksp_B =
A;
562 fix_dofs_fe->ksp_B =
A;
566 fix_dofs_fe->ksp_f =
f;
567 pressure_fe->ksp_f =
f;
583 CHKERR KSPCreate(PETSC_COMM_WORLD, &solver);
587 CHKERR KSPSetFromOptions(solver);
590 CHKERR KSPSetOperators(solver,
A,
A);
599 CHKERR KSPDestroy(&solver);
603 VecView(x, PETSC_VIEWER_STDOUT_WORLD);
610 auto get_post_proc_ele = [&]() {
611 auto jac_ptr = boost::make_shared<MatrixDouble>();
612 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
613 auto det_ptr = boost::make_shared<VectorDouble>();
615 auto post_proc_ele = boost::make_shared<
619 post_proc_ele->getOpPtrVector().push_back(
621 post_proc_ele->getOpPtrVector().push_back(
623 post_proc_ele->getOpPtrVector().push_back(
626 auto u_ptr = boost::make_shared<MatrixDouble>();
627 auto grad_ptr = boost::make_shared<MatrixDouble>();
628 post_proc_ele->getOpPtrVector().push_back(
630 post_proc_ele->getOpPtrVector().push_back(
635 post_proc_ele->getOpPtrVector().push_back(
639 post_proc_ele->getPostProcMesh(), post_proc_ele->getMapGaussPts(),
644 {{
"GRAD", grad_ptr}},
650 return post_proc_ele;
653 if (
auto post_proc = get_post_proc_ele()) {
657 CHKERR post_proc->writeFile(
"out.h5m");
661 if (flg_test == PETSC_TRUE) {
662 const double x_vec_norm_const = 0.4;
667 CHKERR VecNorm(x, NORM_INFINITY, &norm_check);
668 if (std::abs(norm_check - x_vec_norm_const) / x_vec_norm_const >
671 "test failed (nrm 2 %6.4e)", norm_check);
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
FormsIntegrators< DomainEleOp >::Assembly< A >::BiLinearForm< I >::OpGradSymTensorGrad< BASE_DIM, SPACE_DIM, SPACE_DIM, 0 > OpK
[Define entities]
PetscErrorCode DMMoFEMKSPSetComputeRHS(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set KSP right hand side evaluation function
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
MoFEMErrorCode buildProblem()
Build problem.
Get values at integration pts for tensor filed rank 1, i.e. vector field.
#define _IT_CUBITMESHSETS_BY_BCDATA_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet in a moFEM field.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
Set integration rule to volume elements.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
PetscErrorCode DMMoFEMKSPSetComputeOperators(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
Set KSP operators and push mofem finite element methods.
Simple interface for fast problem set-up.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
Deprecated interface functions.
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
Set inverse jacobian to base functions.
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
PetscErrorCode DMoFEMMeshToGlobalVector(DM dm, Vec g, InsertMode mode, ScatterMode scatter_mode)
set ghosted vector values on all existing mesh entities
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
const std::string getDomainFEName() const
Get the Domain FE Name.
Set integration rule to boundary elements.
PetscErrorCode DMoFEMPostProcessFiniteElements(DM dm, MoFEM::FEMethod *method)
execute finite element method for each element in dm (problem)
virtual MoFEMErrorCode add_ents_to_finite_element_by_dim(const EntityHandle entities, const int dim, const std::string &name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
Volume finite element base.
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
MoFEMErrorCode buildFiniteElements()
Build finite elements.
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const FTensor::Tensor2< T, Dim, Dim > Vec
@ MOFEM_DATA_INCONSISTENCY
MoFEMErrorCode defineFiniteElements()
Define finite elements.
MoFEMErrorCode buildFields()
Build fields.
@ MOFEM_ATOM_TEST_INVALID
MoFEMErrorCode defineProblem(const PetscBool is_partitioned=PETSC_TRUE)
define problem
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
PetscErrorCode DMMoFEMSetIsPartitioned(DM dm, PetscBool is_partitioned)
Post post-proc data at points from hash maps.