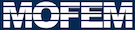 |
| v0.14.0
|
Definition at line 10 of file nonlinear_poisson_2d.cpp.
◆ PostProcEle
◆ NonlinearPoisson()
◆ assembleSystem()
Definition at line 198 of file nonlinear_poisson_2d.cpp.
201 auto det_ptr = boost::make_shared<VectorDouble>();
202 auto jac_ptr = boost::make_shared<MatrixDouble>();
203 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
284 boost::shared_ptr<FaceEle> null_face;
293 boost::shared_ptr<EdgeEle> null_edge;
◆ boundaryCondition()
Definition at line 157 of file nonlinear_poisson_2d.cpp.
161 auto get_ents_on_mesh_skin = [&]() {
162 Range boundary_entities;
164 std::string entity_name = it->getName();
165 if (entity_name.compare(0, 18,
"BOUNDARY_CONDITION") == 0) {
167 boundary_entities,
true);
171 Range boundary_vertices;
173 boundary_vertices,
true);
174 boundary_entities.merge(boundary_vertices);
179 return boundary_entities;
182 auto mark_boundary_dofs = [&](
Range &&skin_edges) {
184 auto marker_ptr = boost::make_shared<std::vector<unsigned char>>();
186 skin_edges, *marker_ptr);
◆ boundaryFunction()
◆ outputResults()
◆ readMesh()
◆ runProgram()
◆ setIntegrationRules()
Definition at line 141 of file nonlinear_poisson_2d.cpp.
144 auto domain_rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * (p + 1); };
145 auto domain_rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * (p + 1); };
149 auto boundary_rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * p + 1; };
150 auto boundary_rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * p + 1; };
◆ setupProblem()
◆ solveSystem()
Definition at line 305 of file nonlinear_poisson_2d.cpp.
322 CHKERR KSPGetPC(ksp_solver, &pc);
324 PetscBool is_pcfs = PETSC_FALSE;
325 PetscObjectTypeCompare((PetscObject)pc, PCFIELDSPLIT, &is_pcfs);
328 if (is_pcfs == PETSC_TRUE) {
337 CHKERR PCFieldSplitSetIS(pc, NULL, is_boundary);
339 CHKERR ISDestroy(&is_boundary);
◆ sourceTermFunction()
◆ boundaryEntitiesForFieldsplit
Range NonlinearPoisson::boundaryEntitiesForFieldsplit |
|
private |
◆ boundaryMarker
boost::shared_ptr<std::vector<unsigned char> > NonlinearPoisson::boundaryMarker |
|
private |
◆ boundaryResidualVectorPipeline
boost::shared_ptr<EdgeEle> NonlinearPoisson::boundaryResidualVectorPipeline |
|
private |
◆ boundaryTangentMatrixPipeline
boost::shared_ptr<EdgeEle> NonlinearPoisson::boundaryTangentMatrixPipeline |
|
private |
◆ dM
◆ domainField
std::string NonlinearPoisson::domainField |
|
private |
◆ domainResidualVectorPipeline
boost::shared_ptr<FaceEle> NonlinearPoisson::domainResidualVectorPipeline |
|
private |
◆ domainTangentMatrixPipeline
boost::shared_ptr<FaceEle> NonlinearPoisson::domainTangentMatrixPipeline |
|
private |
◆ fieldGradPtr
boost::shared_ptr<MatrixDouble> NonlinearPoisson::fieldGradPtr |
|
private |
◆ fieldValuePtr
boost::shared_ptr<VectorDouble> NonlinearPoisson::fieldValuePtr |
|
private |
◆ mField
◆ mpiComm
MPI_Comm NonlinearPoisson::mpiComm |
|
private |
◆ mpiRank
const int NonlinearPoisson::mpiRank |
|
private |
◆ order
int NonlinearPoisson::order |
|
private |
◆ postProc
boost::shared_ptr<PostProcEle> NonlinearPoisson::postProc |
|
private |
◆ previousUpdate
◆ simpleInterface
Simple* NonlinearPoisson::simpleInterface |
|
private |
◆ snesSolver
The documentation for this struct was generated from the following file:
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
MoFEMErrorCode outputResults()
Problem manager is used to build and partition problems.
boost::shared_ptr< VectorDouble > fieldValuePtr
static double boundaryFunction(const double x, const double y, const double z)
virtual MPI_Comm & get_comm() const =0
auto createSNES(MPI_Comm comm)
MoFEMErrorCode boundaryCondition()
static double sourceTermFunction(const double x, const double y, const double z)
virtual int get_comm_rank() const =0
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
boost::shared_ptr< DataAtGaussPoints > previousUpdate
boost::shared_ptr< EdgeEle > boundaryTangentMatrixPipeline
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
PetscErrorCode DMCreateGlobalVector_MoFEM(DM dm, Vec *g)
DMShellSetCreateGlobalVector.
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode setIntegrationRules()
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
boost::shared_ptr< std::vector< unsigned char > > boundaryMarker
virtual moab::Interface & get_moab()=0
MoFEMErrorCode assembleSystem()
Section manager is used to create indexes and sections.
boost::shared_ptr< FaceEle > domainResidualVectorPipeline
SmartPetscObj< SNES > snesSolver
MoFEM::EdgeElementForcesAndSourcesCore EdgeEle
MoFEMErrorCode solveSystem()
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
PetscErrorCode DMoFEMMeshToGlobalVector(DM dm, Vec g, InsertMode mode, ScatterMode scatter_mode)
set ghosted vector values on all existing mesh entities
Get value at integration points for scalar field.
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
const std::string getDomainFEName() const
Get the Domain FE Name.
Modify integration weights on face to take in account higher-order geometry.
MoFEMErrorCode markDofs(const std::string problem_name, RowColData rc, const enum MarkOP op, const Range ents, std::vector< unsigned char > &marker) const
Create vector with marked indices.
PetscErrorCode DMMoFEMSNESSetJacobian(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES Jacobian evaluation function
boost::shared_ptr< MatrixDouble > fieldGradPtr
MoFEM::Interface & mField
@ AINSWORTH_BERNSTEIN_BEZIER_BASE
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
MoFEM::FaceElementForcesAndSourcesCore FaceEle
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
#define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet having a particular BC meshset in a moFEM field.
const std::string getBoundaryFEName() const
Get the Boundary FE Name.
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
boost::shared_ptr< FaceEle > domainTangentMatrixPipeline
MoFEMErrorCode addBoundaryField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on boundary.
MoFEMErrorCode setupProblem()
keeps basic data about problem
boost::shared_ptr< EdgeEle > boundaryResidualVectorPipeline
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
const std::string getProblemName() const
Get the Problem Name.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
boost::shared_ptr< PostProcEle > postProc
MoFEMErrorCode readMesh()
Range boundaryEntitiesForFieldsplit
PetscErrorCode DMMoFEMSNESSetFunction(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES residual evaluation function
Post post-proc data at points from hash maps.