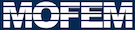 |
| v0.14.0
|
◆ NORMS
◆ Poisson2DNonhomogeneous()
◆ assembleSystem()
[Boundary condition]
[Assemble system]
Definition at line 143 of file poisson_2d_nonhomogeneous.cpp.
151 pipeline_mng->getOpDomainLhsPipeline(), {H1});
152 pipeline_mng->getOpDomainLhsPipeline().push_back(
154 pipeline_mng->getOpDomainLhsPipeline().push_back(
156 pipeline_mng->getOpDomainLhsPipeline().push_back(
162 pipeline_mng->getOpDomainRhsPipeline().push_back(
164 pipeline_mng->getOpDomainRhsPipeline().push_back(
166 pipeline_mng->getOpDomainRhsPipeline().push_back(
172 pipeline_mng->getOpBoundaryLhsPipeline().push_back(
174 pipeline_mng->getOpBoundaryLhsPipeline().push_back(
176 pipeline_mng->getOpBoundaryLhsPipeline().push_back(
182 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
184 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
186 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
◆ boundaryCondition()
[Setup problem]
[Boundary condition]
Definition at line 125 of file poisson_2d_nonhomogeneous.cpp.
133 boundaryMarker = bc_mng->getMergedBlocksMarker({
"BOUNDARY_CONDITION"});
136 bc_mng->getMergedBlocksRange({
"BOUNDARY_CONDITION"});
◆ boundaryFunction()
◆ checkResults()
[Output results]
[Check]
Definition at line 297 of file poisson_2d_nonhomogeneous.cpp.
300 auto check_result_fe_ptr = boost::make_shared<FaceEle>(
mField);
306 check_result_fe_ptr->getOpPtrVector(), {H1})),
309 check_result_fe_ptr->getRuleHook = [](
int,
int,
int p) {
return 2 * p; };
310 auto analyticalFunction = [&](
double x,
double y,
double z) {
311 return cos(x * M_PI) * cos(y * M_PI);
314 auto u_ptr = boost::make_shared<VectorDouble>();
316 check_result_fe_ptr->getOpPtrVector().push_back(
318 auto mValFuncPtr = boost::make_shared<VectorDouble>();
319 check_result_fe_ptr->getOpPtrVector().push_back(
321 check_result_fe_ptr->getOpPtrVector().push_back(
323 CHKERR VecZeroEntries(petscVec);
326 check_result_fe_ptr);
327 CHKERR VecAssemblyBegin(petscVec);
328 CHKERR VecAssemblyEnd(petscVec);
332 CHKERR VecGetArrayRead(petscVec, &norms);
334 <<
"NORM: " << std::sqrt(norms[
NORM]);
335 CHKERR VecRestoreArrayRead(petscVec, &norms);
339 CHKERR VecGetArrayRead(petscVec, &t_ptr);
340 double ref_norm = 1.4e-04;
344 cal_norm = sqrt(t_ptr[0]);
348 "atom test %d does not exist",
atom_test);
350 if (cal_norm > ref_norm) {
352 "atom test %d failed! Calculated Norm %3.16e is greater than "
353 "reference Norm %3.16e",
356 CHKERR VecRestoreArrayRead(petscVec, &t_ptr);
◆ outputResults()
[Solve system]
[Output results]
Definition at line 266 of file poisson_2d_nonhomogeneous.cpp.
271 pipeline_mng->getBoundaryLhsFE().reset();
272 pipeline_mng->getDomainRhsFE().reset();
273 pipeline_mng->getBoundaryRhsFE().reset();
275 auto d_ptr = boost::make_shared<VectorDouble>();
276 auto l_ptr = boost::make_shared<VectorDouble>();
280 auto post_proc_fe = boost::make_shared<PostProcFaceEle>(
mField);
282 post_proc_fe->getOpPtrVector().push_back(
284 post_proc_fe->getOpPtrVector().push_back(
new OpPPMap(
285 post_proc_fe->getPostProcMesh(), post_proc_fe->getMapGaussPts(),
286 {{domainField, d_ptr}}, {}, {}, {}));
287 pipeline_mng->getDomainRhsFE() = post_proc_fe;
289 CHKERR pipeline_mng->loopFiniteElements();
290 CHKERR post_proc_fe->writeFile(
"out_result.h5m");
◆ readMesh()
◆ runProgram()
◆ setIntegrationRules()
[Assemble system]
[Set integration rules]
Definition at line 195 of file poisson_2d_nonhomogeneous.cpp.
200 auto domain_rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * (p - 1); };
201 auto domain_rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * (p - 1); };
202 CHKERR pipeline_mng->setDomainLhsIntegrationRule(domain_rule_lhs);
203 CHKERR pipeline_mng->setDomainRhsIntegrationRule(domain_rule_rhs);
205 auto boundary_rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * p; };
206 auto boundary_rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * p; };
207 CHKERR pipeline_mng->setBoundaryLhsIntegrationRule(boundary_rule_lhs);
208 CHKERR pipeline_mng->setBoundaryLhsIntegrationRule(boundary_rule_rhs);
◆ setupProblem()
[Read mesh]
[Setup problem]
Definition at line 72 of file poisson_2d_nonhomogeneous.cpp.
77 auto get_ents_by_dim = [&](
const auto dim) {
90 auto get_base = [&]() {
91 auto domain_ents = get_ents_by_dim(
SPACE_DIM);
92 if (domain_ents.empty())
109 auto base = get_base();
◆ solveSystem()
[Set integration rules]
[Solve system]
Definition at line 215 of file poisson_2d_nonhomogeneous.cpp.
220 auto ksp_solver = pipeline_mng->
createKSP();
221 CHKERR KSPSetFromOptions(ksp_solver);
231 CHKERR KSPGetPC(ksp_solver, &pc);
232 PetscBool is_pcfs = PETSC_FALSE;
233 PetscObjectTypeCompare((PetscObject)pc, PCFIELDSPLIT, &is_pcfs);
238 if (is_pcfs == PETSC_TRUE) {
239 IS is_domain, is_boundary;
246 CHKERR PCFieldSplitSetIS(pc, NULL, is_boundary);
247 CHKERR ISDestroy(&is_boundary);
251 CHKERR KSPSetUp(ksp_solver);
257 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
258 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
◆ sourceTermFunction()
◆ atom_test
int Poisson2DNonhomogeneous::atom_test = 0 |
|
private |
◆ boundaryEntitiesForFieldsplit
Range Poisson2DNonhomogeneous::boundaryEntitiesForFieldsplit |
|
private |
◆ boundaryMarker
boost::shared_ptr<std::vector<unsigned char> > Poisson2DNonhomogeneous::boundaryMarker |
|
private |
◆ domainField
std::string Poisson2DNonhomogeneous::domainField |
|
private |
◆ mField
◆ oRder
int Poisson2DNonhomogeneous::oRder |
|
private |
◆ simpleInterface
Simple* Poisson2DNonhomogeneous::simpleInterface |
|
private |
The documentation for this struct was generated from the following file:
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
#define MOFEM_LOG_CHANNEL(channel)
Set and reset channel.
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpBaseTimesScalar< 1 > OpBoundaryRhs
MoFEMErrorCode assembleSystem()
[Boundary condition]
virtual MPI_Comm & get_comm() const =0
#define CHK_THROW_MESSAGE(err, msg)
Check and throw MoFEM exception.
virtual int get_comm_rank() const =0
MoFEM::Interface & mField
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
PipelineManager interface.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
MoFEMErrorCode outputResults()
[Solve system]
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
auto createDMVector(DM dm)
Get smart vector from DM.
virtual moab::Interface & get_moab()=0
MoFEMErrorCode readMesh()
[Read mesh]
Section manager is used to create indexes and sections.
Simple interface for fast problem set-up.
static double boundaryFunction(const double x, const double y, const double z)
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
static double sourceTermFunction(const double x, const double y, const double z)
Get value at integration points for scalar field.
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
SmartPetscObj< KSP > createKSP(SmartPetscObj< DM > dm=nullptr)
Create KSP (linear) solver.
const std::string getDomainFEName() const
Get the Domain FE Name.
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, 1 > OpBoundaryLhs
MoFEMErrorCode solveSystem()
[Set integration rules]
auto type_from_handle(const EntityHandle h)
get type from entity handle
MoFEMErrorCode setIntegrationRules()
[Assemble system]
Get values from scalar function at integration points and save them to VectorDouble for Tensor0.
Range boundaryEntitiesForFieldsplit
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
Add operators pushing bases from local to physical configuration.
boost::shared_ptr< FEMethod > & getDomainLhsFE()
#define MOFEM_TAG_AND_LOG(channel, severity, tag)
Tag and log in channel.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
MoFEMErrorCode boundaryCondition()
[Setup problem]
MoFEMErrorCode setupProblem()
[Read mesh]
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
Set indices on entities on finite element.
Get norm of input VectorDouble for Tensor0.
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
auto createVectorMPI(MPI_Comm comm, PetscInt n, PetscInt N)
Create MPI Vector.
MoFEMErrorCode addBoundaryField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on boundary.
boost::shared_ptr< std::vector< unsigned char > > boundaryMarker
const double D
diffusivity
@ MOFEM_ATOM_TEST_INVALID
keeps basic data about problem
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
const std::string getProblemName() const
Get the Problem Name.
MoFEMErrorCode checkResults()
[Output results]
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
Post post-proc data at points from hash maps.