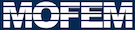 |
| v0.14.0
|
Definition at line 24 of file standard_poisson.cpp.
◆ StandardPoisson()
◆ analyticalFunction()
[Analytical function]
Definition at line 44 of file standard_poisson.cpp.
46 return exp(-100. * (
sqr(x) +
sqr(y))) * cos(M_PI * x) * cos(M_PI * y);
◆ analyticalFunctionGrad()
static VectorDouble StandardPoisson::analyticalFunctionGrad |
( |
const double |
x, |
|
|
const double |
y, |
|
|
const double |
z |
|
) |
| |
|
inlinestaticprivate |
[Analytical function]
[Analytical function gradient]
Definition at line 51 of file standard_poisson.cpp.
55 res[0] = -exp(-100. * (
sqr(x) +
sqr(y))) *
56 (200. * x * cos(M_PI * x) + M_PI * sin(M_PI * x)) * cos(M_PI * y);
57 res[1] = -exp(-100. * (
sqr(x) +
sqr(y))) *
58 (200. * y * cos(M_PI * y) + M_PI * sin(M_PI * y)) * cos(M_PI * x);
◆ assembleSystem()
[Create common data]
Definition at line 175 of file standard_poisson.cpp.
184 pipeline_mng->getOpDomainLhsPipeline().push_back(
190 pipeline_mng->getOpDomainRhsPipeline().push_back(
◆ boundaryCondition()
◆ checkError()
[Check error]
Definition at line 242 of file standard_poisson.cpp.
268 <<
"Global error L2 norm: " << std::sqrt(array[0]);
270 <<
"Global error H1 seminorm: " << std::sqrt(array[1]);
◆ createCommonData()
[Create common data]
Definition at line 159 of file standard_poisson.cpp.
162 PetscInt ghosts[2] = {0, 1};
169 commonDataPtr->approxVals = boost::make_shared<VectorDouble>();
170 commonDataPtr->approxValsGrad = boost::make_shared<MatrixDouble>();
◆ outputResults()
[Check error]
Definition at line 277 of file standard_poisson.cpp.
282 pipeline_mng->getBoundaryLhsFE().reset();
283 pipeline_mng->getDomainRhsFE().reset();
284 pipeline_mng->getBoundaryRhsFE().reset();
288 auto post_proc_fe = boost::make_shared<PostProcFaceEle>(
mField);
292 post_proc_fe->getOpPtrVector().push_back(
294 post_proc_fe->getOpPtrVector().push_back(
298 post_proc_fe->getOpPtrVector().push_back(
new OpPPMap(
299 post_proc_fe->getPostProcMesh(), post_proc_fe->getMapGaussPts(),
300 {{domainField, commonDataPtr->approxVals}},
302 pipeline_mng->getDomainRhsFE() = post_proc_fe;
304 CHKERR pipeline_mng->loopFiniteElements();
305 CHKERR post_proc_fe->writeFile(
"out_result.h5m");
◆ readMesh()
◆ runProgram()
◆ setIntegrationRules()
Definition at line 197 of file standard_poisson.cpp.
202 auto domain_rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * (p - 1); };
203 auto domain_rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * (p - 1); };
204 CHKERR pipeline_mng->setDomainLhsIntegrationRule(domain_rule_lhs);
205 CHKERR pipeline_mng->setDomainRhsIntegrationRule(domain_rule_rhs);
207 auto boundary_rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * p; };
208 auto boundary_rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * p; };
209 CHKERR pipeline_mng->setBoundaryLhsIntegrationRule(boundary_rule_lhs);
210 CHKERR pipeline_mng->setBoundaryLhsIntegrationRule(boundary_rule_rhs);
◆ setupProblem()
◆ solveSystem()
Definition at line 215 of file standard_poisson.cpp.
220 auto ksp_solver = pipeline_mng->
createKSP();
221 CHKERR KSPSetFromOptions(ksp_solver);
228 CHKERR KSPSetUp(ksp_solver);
234 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
235 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
◆ sourceFunction()
[Analytical function gradient]
[Source function]
Definition at line 64 of file standard_poisson.cpp.
65 return -exp(-100. * (
sqr(x) +
sqr(y))) *
67 (x * cos(M_PI * y) * sin(M_PI * x) +
68 y * cos(M_PI * x) * sin(M_PI * y)) +
69 2. * (20000. * (
sqr(x) +
sqr(y)) - 200. -
sqr(M_PI)) *
70 cos(M_PI * x) * cos(M_PI * y));
◆ boundaryEntitiesForFieldsplit
Range StandardPoisson::boundaryEntitiesForFieldsplit |
|
private |
◆ boundaryMarker
boost::shared_ptr<std::vector<unsigned char> > StandardPoisson::boundaryMarker |
|
private |
◆ commonDataPtr
boost::shared_ptr<CommonData> StandardPoisson::commonDataPtr |
|
private |
◆ domainField
std::string StandardPoisson::domainField |
|
private |
◆ mField
◆ oRder
int StandardPoisson::oRder |
|
private |
◆ simpleInterface
Simple* StandardPoisson::simpleInterface |
|
private |
The documentation for this struct was generated from the following file:
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
boost::shared_ptr< FEMethod > & getDomainRhsFE()
virtual MPI_Comm & get_comm() const =0
MoFEMErrorCode loopFiniteElements(SmartPetscObj< DM > dm=nullptr)
Iterate finite elements.
boost::ptr_deque< UserDataOperator > & getOpDomainRhsPipeline()
Get the Op Domain Rhs Pipeline object.
virtual int get_comm_rank() const =0
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
PipelineManager interface.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
auto createDMVector(DM dm)
Get smart vector from DM.
auto createGhostVector(MPI_Comm comm, PetscInt n, PetscInt N, PetscInt nghost, const PetscInt ghosts[])
Create smart ghost vector.
MoFEM::Interface & mField
Simple interface for fast problem set-up.
MoFEMErrorCode setupProblem()
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
Get value at integration points for scalar field.
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
SmartPetscObj< KSP > createKSP(SmartPetscObj< DM > dm=nullptr)
Create KSP (linear) solver.
static double sourceFunction(const double x, const double y, const double z)
[Analytical function gradient]
MoFEMErrorCode readMesh()
MoFEMErrorCode outputResults()
[Check error]
MoFEMErrorCode setIntegrationRules()
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
Add operators pushing bases from local to physical configuration.
MoFEMErrorCode checkError()
[Check error]
boost::shared_ptr< FEMethod > & getDomainLhsFE()
#define MOFEM_LOG(channel, severity)
Log.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
boost::shared_ptr< CommonData > commonDataPtr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
MoFEMErrorCode solveSystem()
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpGradGrad< 1, 1, SPACE_DIM > OpDomainGradGrad
UBlasVector< double > VectorDouble
MoFEMErrorCode addBoundaryField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on boundary.
const double D
diffusivity
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
const std::string getProblemName() const
Get the Problem Name.
MoFEMErrorCode assembleSystem()
[Create common data]
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode createCommonData()
[Create common data]
MoFEMErrorCode boundaryCondition()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, 1 > OpDomainSource
Post post-proc data at points from hash maps.