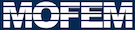 |
| v0.14.0
|
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
|
static char | help [] = "...\n\n" |
|
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
Definition at line 14 of file build_composite_problem.cpp.
20 PetscBool flg = PETSC_TRUE;
22 #if PETSC_VERSION_GE(3, 6, 4)
29 if (flg != PETSC_TRUE) {
30 SETERRQ(PETSC_COMM_SELF, 1,
"*** ERROR -my_file (MESH FILE NEEDED)");
47 CHKERR moab.get_entities_by_type(root_set, MBTET, tets,
false);
51 CHKERR comm_interface_ptr->partitionMesh(
55 CHKERR moab.create_meshset(MESHSET_SET, part_set);
57 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
60 "Communicator should be allocated");
62 Tag part_tag = pcomm->part_tag();
66 0, MBENTITYSET, &part_tag, NULL, 1, tagged_sets,
67 moab::Interface::UNION);
68 for (Range::iterator mit = tagged_sets.begin(); mit != tagged_sets.end();
71 CHKERR moab.tag_get_data(part_tag, &*mit, 1, &part);
74 CHKERR moab.get_entities_by_type(*mit, MBTET, proc_ents,
true);
75 CHKERR moab.add_entities(part_set, proc_ents);
81 CHKERR skin.find_skin(0, tets,
false, tets_skin);
82 Range proc_ents_skin[4];
83 proc_ents_skin[3] = proc_ents;
84 CHKERR skin.find_skin(0, proc_ents,
false, proc_ents_skin[2]);
85 proc_ents_skin[2] = subtract(proc_ents_skin[2], tets_skin);
86 CHKERR moab.get_adjacencies(proc_ents_skin[2], 1,
false, proc_ents_skin[1],
87 moab::Interface::UNION);
88 CHKERR moab.get_connectivity(proc_ents_skin[1], proc_ents_skin[0],
true);
89 for (
int dd = 0;
dd != 3;
dd++) {
90 CHKERR moab.add_entities(part_set, proc_ents_skin[
dd]);
94 std::ostringstream file_skin;
95 file_skin <<
"out_skin_" << m_field.
get_comm_rank() <<
".vtk";
97 CHKERR moab.create_meshset(MESHSET_SET, meshset_skin);
98 CHKERR moab.add_entities(meshset_skin, proc_ents_skin[2]);
99 CHKERR moab.add_entities(meshset_skin, proc_ents_skin[1]);
100 CHKERR moab.add_entities(meshset_skin, proc_ents_skin[0]);
101 CHKERR moab.write_file(file_skin.str().c_str(),
"VTK",
"", &meshset_skin,
105 CHKERR pcomm->resolve_shared_ents(0, proc_ents, 3, -1, proc_ents_skin);
106 Range owned_tets = proc_ents;
109 std::ostringstream file_owned;
110 file_owned <<
"out_owned_" << m_field.
get_comm_rank() <<
".vtk";
112 CHKERR moab.create_meshset(MESHSET_SET, meshset_owned);
113 CHKERR moab.add_entities(meshset_owned, owned_tets);
114 CHKERR moab.write_file(file_owned.str().c_str(),
"VTK",
"",
119 CHKERR pcomm->get_shared_entities(-1, shared_ents);
122 std::ostringstream file_shared_owned;
123 file_shared_owned <<
"out_shared_owned_" << m_field.
get_comm_rank()
126 CHKERR moab.create_meshset(MESHSET_SET, meshset_shared_owned);
127 CHKERR moab.add_entities(meshset_shared_owned, shared_ents);
128 CHKERR moab.write_file(file_shared_owned.str().c_str(),
"VTK",
"",
129 &meshset_shared_owned, 1);
136 part_set, 3, bit_level0);
189 ->createMPIAIJWithArrays<PetscGlobalIdx_mi_tag>(
"P1",
m);
190 MatView(
m, PETSC_VIEWER_DRAW_WORLD);
196 ->checkMPIAIJWithArraysMatrixFillIn<PetscGlobalIdx_mi_tag>(
"P1", -1, -1,
199 ->checkMPIAIJWithArraysMatrixFillIn<PetscGlobalIdx_mi_tag>(
"P2", -1, -1,
203 DMType dm_name =
"MOFEM";
209 CHKERR DMSetFromOptions(dm);
220 ->createMPIAIJWithArrays<PetscGlobalIdx_mi_tag>(
"COMP",
m);
221 MatView(
m, PETSC_VIEWER_DRAW_WORLD);
227 ->checkMPIAIJWithArraysMatrixFillIn<PetscGlobalIdx_mi_tag>(
"COMP", -1,
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
#define MYPCOMM_INDEX
default communicator number PCOMM
Problem manager is used to build and partition problems.
virtual MPI_Comm & get_comm() const =0
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
virtual int get_comm_rank() const =0
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
Deprecated interface functions.
DeprecatedCoreInterface Interface
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string &name, const bool recursive=true)=0
add entities to finite element
PetscErrorCode DMMoFEMAddRowCompositeProblem(DM dm, const char prb_name[])
Add problem to composite DM on row.
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
auto createDM(MPI_Comm comm, const std::string dm_type_name)
Creates smart DM object.
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
virtual int get_comm_size() const =0
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
Matrix manager is used to build and partition problems.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
const Tensor2_symmetric_Expr< const ddTensor0< T, Dim, i, j >, typename promote< T, double >::V, Dim, i, j > dd(const Tensor0< T * > &a, const Index< i, Dim > index1, const Index< j, Dim > index2, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
@ MOFEM_DATA_INCONSISTENCY
MoFEMErrorCode buildProblemOnDistributedMesh(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures, assuming that mesh is distributed (collective)
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
FTensor::Index< 'm', 3 > m
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
PetscErrorCode DMMoFEMSetIsPartitioned(DM dm, PetscBool is_partitioned)