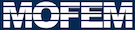 |
| v0.14.0
|
Go to the documentation of this file.
11 static char help[] =
"...\n\n";
41 int main(
int argc,
char *argv[]) {
47 PetscBool flg = PETSC_TRUE;
49 #if PETSC_VERSION_GE(3, 6, 4)
56 if (flg != PETSC_TRUE) {
57 SETERRQ(PETSC_COMM_SELF, 1,
"*** ERROR -my_file (MESH FILE NEEDED)");
61 DMType dm_name =
"DMMOFEM";
66 CHKERR DMCreate(PETSC_COMM_WORLD, &dm);
67 CHKERR DMSetType(dm, dm_name);
73 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
75 boost::make_shared<WrapMPIComm>(PETSC_COMM_WORLD,
false);
77 pcomm =
new ParallelComm(&moab, moab_comm_wrap->get_comm());
80 option =
"PARALLEL=BCAST_DELETE;PARALLEL_RESOLVE_SHARED_ENTS;PARTITION="
81 "PARALLEL_PARTITION;";
92 root_set, 3, bit_level0);
95 #if PETSC_VERSION_GE(3, 6, 4)
150 CHKERR DMSetFromOptions(dm);
160 ->checkMPIAIJWithArraysMatrixFillIn<PetscGlobalIdx_mi_tag>(
161 "TEST_PROBLEM", -1, -1, 1);
164 ->checkMPIAIJMatrixFillIn<PetscGlobalIdx_mi_tag>(
"TEST_PROBLEM", -1, -1,
167 std::vector<std::string> fields_list;
168 fields_list.push_back(
"FIELD1");
171 PetscSection section;
174 CHKERR PetscSectionView(section, PETSC_VIEWER_STDOUT_WORLD);
175 CHKERR DMSetSection(dm, section);
176 CHKERR PetscSectionDestroy(§ion);
178 PetscBool save_file = PETSC_TRUE;
179 #if PETSC_VERSION_GE(3, 6, 4)
188 CHKERR PetscViewerASCIIOpen(PETSC_COMM_WORLD,
189 "dm_build_partitioned_mesh.txt", &viewer);
190 CHKERR PetscViewerPushFormat(viewer, PETSC_VIEWER_ASCII_INFO);
192 CHKERR PetscViewerDestroy(&viewer);
197 boost::make_shared<CountUp>(count));
199 boost::make_shared<CountDown>(count));
204 auto check_consistency_of_uids = [&]() {
207 for (
auto &fe : *field_ents_ptr) {
208 if (fe->getOwnerProc() !=
215 <<
"Entity owner proc " << fe->getOwnerProc();
217 "UId and entity handle inconsistency");
225 MOFEM_LOG(
"SELF", Sev::error) <<
"Entity handle " << fe->getEnt();
227 "UId and entity handle inconsistency");
229 const auto field_bit_number_from_uid =
231 if (fe->getBitNumber() != field_bit_number_from_uid) {
234 <<
"UId field bit " << field_bit_number_from_uid;
236 <<
"Entity owner proc " << fe->getBitNumber();
238 "UId and entity handle inconsistency");
241 field_bit_number_from_uid - 1))) {
246 BitFieldId().set(field_bit_number_from_uid - 1));
248 <<
"Entity owner proc " << fe->getName();
250 "UId and entity handle inconsistency");
257 CHKERR check_consistency_of_uids();
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
MoFEMErrorCode operator()()
function is run for every finite element
virtual std::string get_field_name(const BitFieldId id) const =0
get field name from id
#define MYPCOMM_INDEX
default communicator number PCOMM
MoFEMErrorCode operator()()
function is run for every finite element
structure for User Loop Methods on finite elements
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
PetscErrorCode DMMoFEMSetSquareProblem(DM dm, PetscBool square_problem)
set squared problem
@ L2
field with C-1 continuity
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
std::bitset< BITFIELDID_SIZE > BitFieldId
Field Id.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
Deprecated interface functions.
DeprecatedCoreInterface Interface
MoFEMErrorCode postProcess()
function is run at the end of loop
PetscErrorCode DMCreateMatrix_MoFEM(DM dm, Mat *M)
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
Section manager is used to create indexes and sections.
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
MoFEMErrorCode preProcess()
function is run at the beginning of loop
static auto getHandleFromUniqueId(const UId uid)
Get the Handle From Unique Id.
int main(int argc, char *argv[])
MoFEMErrorCode preProcess()
function is run at the beginning of loop
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
virtual const FieldEntity_multiIndex * get_field_ents() const =0
Get the field ents object.
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
Matrix manager is used to build and partition problems.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
@ MOFEM_DATA_INCONSISTENCY
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
FTensor::Index< 'm', 3 > m
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
@ MOFEM_ATOM_TEST_INVALID
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
static auto getFieldBitNumberFromUniqueId(const UId uid)
Get the Field Bit Number From Unique Id.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode postProcess()
function is run at the end of loop
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
PetscErrorCode DMMoFEMSetIsPartitioned(DM dm, PetscBool is_partitioned)
PetscErrorCode PetscOptionsGetBool(PetscOptions *, const char pre[], const char name[], PetscBool *bval, PetscBool *set)
static auto getOwnerFromUniqueId(const UId uid)