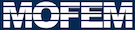 |
| v0.14.0
|
Go to the documentation of this file.
12 using namespace MoFEM;
14 static char help[] =
"...\n\n";
16 #define HelloFunctionBegin \
18 MOFEM_LOG_CHANNEL("SYNC"); \
19 MOFEM_LOG_FUNCTION(); \
20 MOFEM_LOG_TAG("WORLD", "HelloWorld");
28 if (
type == MBVERTEX) {
30 MOFEM_LOG(
"SYNC", Sev::inform) <<
"**** " << getNinTheLoop() <<
" ****";
31 MOFEM_LOG(
"SYNC", Sev::inform) <<
"**** Operators ****";
34 <<
"Hello Operator OpRow:"
35 <<
" field name " << rowFieldName <<
" side " << side <<
" type "
36 << CN::EntityTypeName(
type) <<
" nb dofs on entity "
43 OpRowCol(
const std::string row_field,
const std::string col_field,
53 <<
"Hello Operator OpRowCol:"
54 <<
" row field name " << rowFieldName <<
" row side " << row_side
55 <<
" row type " << CN::EntityTypeName(row_type)
56 <<
" nb dofs on row entity " << row_data.
getIndices().size() <<
" : "
57 <<
" col field name " << colFieldName <<
" col side " << col_side
58 <<
" col type " << CN::EntityTypeName(col_type)
59 <<
" nb dofs on col entity " << col_data.
getIndices().size();
72 if (
type == MBVERTEX) {
73 MOFEM_LOG(
"SYNC", Sev::inform) <<
"Hello Operator OpVolume:"
74 <<
" volume " << getVolume();
86 if (
type == MBVERTEX) {
87 MOFEM_LOG(
"SYNC", Sev::inform) <<
"Hello Operator OpFace:"
88 <<
" normal " << getNormal();
95 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide> &
feSidePtr;
98 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide> &fe_side_ptr)
100 feSidePtr(fe_side_ptr) {}
105 if (
type == MBVERTEX) {
106 MOFEM_LOG(
"SYNC", Sev::inform) <<
"Hello Operator OpSideFace";
107 CHKERR loopSideVolumes(
"dFE", *feSidePtr);
121 if (
type == MBVERTEX) {
123 <<
"Hello Operator OpVolumeSide:"
124 <<
" volume " << getVolume() <<
" normal " << getNormal();
130 int main(
int argc,
char *argv[]) {
138 DMType dm_name =
"DMMOFEM";
173 pipeline_mng->getOpDomainRhsPipeline().push_back(
new OpVolume(
"U"));
174 pipeline_mng->getOpDomainLhsPipeline().push_back(
179 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
new OpRow(
"L"));
180 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
new OpFace(
"L"));
181 pipeline_mng->getOpBoundaryLhsPipeline().push_back(
186 boost::shared_ptr<VolumeElementForcesAndSourcesCoreOnSide> side_fe(
188 side_fe->getOpPtrVector().push_back(
new OpVolumeSide(
"U"));
192 pipeline_mng->getOpSkeletonRhsPipeline().push_back(
new OpRow(
"S"));
193 pipeline_mng->getOpSkeletonRhsPipeline().push_back(
198 CHKERR pipeline_mng->loopFiniteElements();
default operator for TET element
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Data on single entity (This is passed as argument to DataOperator::doWork)
OpFaceSide(const std::string &field_name, boost::shared_ptr< VolumeElementForcesAndSourcesCoreOnSide > &fe_side_ptr)
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
#define HelloFunctionBegin
virtual MPI_Comm & get_comm() const =0
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
OpVolume(const std::string &field_name)
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
OpRow(const std::string &field_name)
boost::ptr_deque< UserDataOperator > & getOpDomainRhsPipeline()
Get the Op Domain Rhs Pipeline object.
PipelineManager interface.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
OpVolumeSide(const std::string &field_name)
default operator for TRI element
void simple(double P1[], double P2[], double P3[], double c[], const int N)
#define MOFEM_LOG_SYNCHRONISE(comm)
Synchronise "SYNC" channel.
const VectorInt & getIndices() const
Get global indices of dofs on entity.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
MoFEMErrorCode doWork(int row_side, int col_side, EntityType row_type, EntityType col_type, EntitiesFieldData::EntData &row_data, EntitiesFieldData::EntData &col_data)
Operator for bi-linear form, usually to calculate values on left hand side.
Volume finite element base.
constexpr auto field_name
OpRowCol(const std::string row_field, const std::string col_field, const bool symm)
structure to get information form mofem into EntitiesFieldData
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
int main(int argc, char *argv[])
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
OpFace(const std::string &field_name)
Base volume element used to integrate on skeleton.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
boost::shared_ptr< VolumeElementForcesAndSourcesCoreOnSide > & feSidePtr