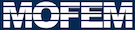 |
| v0.14.0
|
Go to the documentation of this file.
5 namespace bio = boost::iostreams;
11 static char help[] =
"...\n\n";
18 std::vector<VectorDouble> &
operator()(
double x,
double y,
double z) {
28 int main(
int argc,
char *argv[]) {
37 MPI_Comm_rank(PETSC_COMM_WORLD, &rank);
39 PetscBool flg = PETSC_TRUE;
43 if (flg != PETSC_TRUE) {
45 "*** ERROR -my_file (MESH FILE NEEDED)");
60 CHKERR moab.create_meshset(MESHSET_SET, meshset_level0);
80 "MESH_NODE_POSITIONS");
97 "MESH_NODE_POSITIONS");
109 if (flg != PETSC_TRUE) {
130 "MESH_NODE_POSITIONS");
153 ->createMPIAIJWithArrays<PetscGlobalIdx_mi_tag>(
"TEST_PROBLEM", &
A);
160 std::vector<Vec> vec_F;
167 "TEST_PROBLEM",
"TEST_FE",
"FIELD1",
A, vec_F, function_evaluator);
170 CHKERR MatAssemblyBegin(
A, MAT_FINAL_ASSEMBLY);
171 CHKERR MatAssemblyEnd(
A, MAT_FINAL_ASSEMBLY);
172 CHKERR VecGhostUpdateBegin(
F, ADD_VALUES, SCATTER_REVERSE);
173 CHKERR VecGhostUpdateEnd(
F, ADD_VALUES, SCATTER_REVERSE);
174 CHKERR VecGhostUpdateBegin(
F, INSERT_VALUES, SCATTER_FORWARD);
175 CHKERR VecGhostUpdateEnd(
F, INSERT_VALUES, SCATTER_FORWARD);
178 CHKERR KSPCreate(PETSC_COMM_WORLD, &solver);
179 CHKERR KSPSetOperators(solver,
A,
A);
181 CHKERR PetscOptionsInsertString(NULL,
182 "-ksp_monitor -ksp_type fgmres -pc_type "
183 "bjacobi -ksp_atol 0 -ksp_rtol 1e-12");
185 CHKERR KSPSetFromOptions(solver);
186 CHKERR PetscOptionsView(NULL, PETSC_VIEWER_STDOUT_WORLD);
189 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
190 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
193 "TEST_PROBLEM",
COL,
D, INSERT_VALUES, SCATTER_REVERSE);
195 CHKERR KSPDestroy(&solver);
202 CHKERR moab.get_entities_by_type(fe_meshset, MBTET, tets,
true);
204 CHKERR moab.get_adjacencies(tets, 1,
false, tets_edges,
205 moab::Interface::UNION);
207 CHKERR moab.create_meshset(MESHSET_SET, edges_meshset);
208 CHKERR moab.add_entities(edges_meshset, tets);
209 CHKERR moab.add_entities(edges_meshset, tets_edges);
210 CHKERR moab.convert_entities(edges_meshset,
true,
false,
false);
213 m_field,
"FIELD1",
true,
false,
"FIELD1");
215 ent_method_field1_on_10nodeTet.
setNodes =
false;
227 typedef tee_device<std::ostream, std::ofstream>
TeeDevice;
230 std::ofstream ofs(
"field_approximation.txt");
235 CHKERR moab.get_entities_by_type(0, MBVERTEX, nodes,
true);
237 nodes_vals.resize(nodes.size(), 3);
238 CHKERR moab.tag_get_data(ent_method_field1_on_10nodeTet.
th, nodes,
239 &*nodes_vals.data().begin());
241 const double eps = 1e-4;
243 my_split.precision(3);
244 my_split.setf(std::ios::fixed);
245 for (DoubleAllocator::iterator it = nodes_vals.data().begin();
246 it != nodes_vals.data().end(); it++) {
247 *it = fabs(*it) <
eps ? 0.0 : *it;
249 my_split << nodes_vals << std::endl;
253 std::map<EntityHandle, double> m0, m1, m2;
256 my_split.precision(3);
257 my_split.setf(std::ios::fixed);
258 double val = fabs(dit->get()->getFieldData()) <
eps
260 : dit->get()->getFieldData();
261 my_split << dit->get()->getPetscGlobalDofIdx() <<
" " << val << std::endl;
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
virtual EntityHandle get_finite_element_meshset(const std::string name) const =0
Problem manager is used to build and partition problems.
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
Finite element for approximating analytical filed on the mesh.
UBlasMatrix< double > MatrixDouble
virtual const Problem * get_problem(const std::string problem_name) const =0
Get the problem object.
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
DeprecatedCoreInterface Interface
Example approx. function.
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
std::vector< VectorDouble > result
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
Vector manager is used to create vectors \mofem_vectors.
MoFEMErrorCode loopMatrixAndVectorVolume(const std::string &problem_name, const std::string &fe_name, const std::string &field_name, Mat A, std::vector< Vec > &vec_F, FUNEVAL &function_evaluator)
assemble matrix and vector
std::vector< VectorDouble > & operator()(double x, double y, double z)
Matrix manager is used to build and partition problems.
#define _IT_NUMEREDDOF_ROW_FOR_LOOP_(PROBLEMPTR, IT)
use with loops to iterate row DOFs
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
int main(int argc, char *argv[])
MoFEMErrorCode partitionSimpleProblem(const std::string name, int verb=VERBOSE)
partition problem dofs
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const FTensor::Tensor2< T, Dim, Dim > Vec
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
const double D
diffusivity
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
keeps basic data about problem
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.