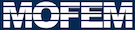 |
| v0.14.0
|
Go to the documentation of this file.
12 using namespace MoFEM;
14 static char help[] =
"...\n\n";
16 int main(
int argc,
char *argv[]) {
25 MPI_Comm_rank(PETSC_COMM_WORLD, &rank);
28 PetscBool flg = PETSC_TRUE;
30 #if PETSC_VERSION_GE(3, 6, 4)
37 if (flg != PETSC_TRUE) {
38 SETERRQ(PETSC_COMM_SELF, 1,
"*** ERROR -my_file (MESH FILE NEEDED)");
41 #if PETSC_VERSION_GE(3, 6, 4)
94 auto check_axpy = [&]() {
97 CHKERR fb->setField(+1, MBVERTEX,
"FIELD_A");
98 CHKERR fb->setField(-2, MBVERTEX,
"FIELD_B");
101 CHKERR fb->fieldAxpy(+0.5,
"FIELD_B",
"FIELD_A");
103 CHKERR fb->fieldScale(-0.5,
"FIELD_B");
106 for (
auto dit : *dofs_ptr) {
108 auto check = [&](
const std::string name,
const double expected) {
110 if (dit->getName() == name) {
111 cout << name <<
" " << dit->getFieldData() <<
" " << expected
113 if (dit->getFieldData() != expected)
115 "Wrong DOF value 0 != %4.3e for %s", dit->getFieldData(),
116 boost::lexical_cast<std::string>(*dit).c_str());
122 CHKERR check(
"FIELD_A", 0);
123 CHKERR check(
"FIELD_B", 1);
128 auto check_set_vertex = [&]() {
131 auto set_distance = [&](
VectorAdaptor &&field_data,
double *xcoord,
132 double *ycoord,
double *zcoord) {
136 xcoord, ycoord, zcoord);
137 field_data[0] = sqrt(t_coord(
i) * t_coord(
i));
141 CHKERR fb->setVertexDofs(set_distance,
"FIELD_A");
150 if (entPtr->getEntType() == MBVERTEX) {
153 CHKERR moab.get_coords(&
v, 1, &coords[0]);
154 if (std::abs(entPtr->getEntFieldData()[0] - norm_2(coords)) >
155 std::numeric_limits<double>::epsilon())
157 "Wrong vals %3.4e != %3.4e",
158 entPtr->getEntFieldData()[0], norm_2(coords));
168 TestMethod method(moab);
175 auto check_lambda = [&]() {
181 if (it->getMeshsetId() != 1)
184 CHKERR it->getMeshsetIdEntitiesByType(m_field.
get_moab(), MBTRI, ents,
187 meshset_ents.merge(nodes);
190 CHKERR fb->setField(+1, MBVERTEX,
"FIELD_A");
191 CHKERR fb->setField(-1, MBVERTEX,
"FIELD_B");
192 CHKERR fb->setField(1.5, MBVERTEX,
"FIELD_C");
194 auto field_axpy = [&](
const double val_y,
const double val_x) {
195 return 0.5 * val_x + val_y;
197 auto field_scale = [&](
const double val) {
return -0.5 * val; };
201 auto vector_times_scalar_field =
202 [&](boost::shared_ptr<FieldEntity> ent_ptr_y,
203 boost::shared_ptr<FieldEntity> ent_ptr_x) {
205 auto x_data = ent_ptr_x->getEntFieldData();
206 auto y_data = ent_ptr_y->getEntFieldData();
208 const auto size_y = y_data.size();
210 for (
size_t dd = 0;
dd != size_y; ++
dd)
211 y_data[
dd] *= x_data[0];
220 CHKERR fb->fieldLambdaOnValues(field_axpy,
"FIELD_B",
"FIELD_A",
true);
225 CHKERR fb->fieldLambdaOnValues(field_scale,
"FIELD_B", &meshset_ents);
228 CHKERR fb->fieldLambdaOnEntities(vector_times_scalar_field,
"FIELD_C",
229 "FIELD_B",
true, &meshset_ents);
232 constexpr
double eps = std::numeric_limits<double>::epsilon();
233 for (
auto dit : *dofs_ptr) {
234 auto check = [&](
const std::string name,
const double expected) {
236 if (dit->getName() == name && dit->getEntType() == MBVERTEX) {
237 cout << name <<
" " << dit->getFieldData() <<
" " << expected
239 if (abs(dit->getFieldData() - expected) >
eps)
241 "Wrong DOF value %4.3e != %4.3e for %s",
242 dit->getFieldData(), expected,
243 boost::lexical_cast<std::string>(*dit).c_str());
249 CHKERR check(
"FIELD_A", 0.5);
251 if (meshset_ents.find(dit->getEnt()) != meshset_ents.end()) {
252 CHKERR check(
"FIELD_B", 0.75);
253 CHKERR check(
"FIELD_C", 1.5);
261 CHKERR check_set_vertex();
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
VectorBoundedArray< double, 3 > VectorDouble3
Data structure to exchange data between mofem and User Loop Methods on entities.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
#define _IT_CUBITMESHSETS_BY_BCDATA_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet in a moFEM field.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
virtual const DofEntity_multiIndex * get_dofs() const =0
Get the dofs object.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
virtual moab::Interface & get_moab()=0
implementation of Data Operators for Forces and Sources
VectorShallowArrayAdaptor< double > VectorAdaptor
FTensor::Index< 'i', SPACE_DIM > i
const double v
phase velocity of light in medium (cm/ns)
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
const Tensor2_symmetric_Expr< const ddTensor0< T, Dim, i, j >, typename promote< T, double >::V, Dim, i, j > dd(const Tensor0< T * > &a, const Index< i, Dim > index1, const Index< j, Dim > index2, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
int main(int argc, char *argv[])
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
@ MOFEM_ATOM_TEST_INVALID
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
virtual MoFEMErrorCode loop_entities(const std::string field_name, EntityMethod &method, Range const *const ents=nullptr, int verb=DEFAULT_VERBOSITY)=0
Loop over field entities.