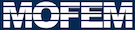 |
| v0.14.0
|
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
- Examples
- prism_polynomial_approximation.cpp.
Definition at line 96 of file prism_polynomial_approximation.cpp.
105 ParallelComm *pcomm = ParallelComm::get_pcomm(&moab,
MYPCOMM_INDEX);
106 auto moab_comm_wrap =
107 boost::make_shared<WrapMPIComm>(PETSC_COMM_WORLD,
false);
109 pcomm =
new ParallelComm(&moab, moab_comm_wrap->get_comm());
111 std::array<double, 18> one_prism_coords = {0, 0, 0, 1, 0, 0, 0, 1, 0,
112 0, 0, 1, 1, 0, 1, 0, 1, 1};
113 std::array<EntityHandle, 6> one_prism_nodes;
114 for (
int n = 0;
n != 6; ++
n)
115 CHKERR moab.create_vertex(&one_prism_coords[3 *
n], one_prism_nodes[
n]);
117 CHKERR moab.create_element(MBPRISM, one_prism_nodes.data(), 6, one_prism);
118 Range one_prism_range;
119 one_prism_range.insert(one_prism);
120 Range one_prism_adj_ents;
121 for (
int d = 1;
d != 3; ++
d)
122 CHKERR moab.get_adjacencies(one_prism_range,
d,
true, one_prism_adj_ents,
123 moab::Interface::UNION);
133 one_prism_range, bit_level0);
183 ->createMPIAIJWithArrays<PetscGlobalIdx_mi_tag>(
"TEST_PROBLEM",
A);
191 auto assemble_matrices_and_vectors = [&]() {
195 fe.getOpPtrVector().push_back(
197 fe.getOpPtrVector().push_back(
199 fe.getOpPtrVector().push_back(
208 CHKERR MatAssemblyBegin(
A, MAT_FINAL_ASSEMBLY);
209 CHKERR MatAssemblyEnd(
A, MAT_FINAL_ASSEMBLY);
213 auto solve_problem = [&] {
215 auto solver =
createKSP(PETSC_COMM_WORLD);
216 CHKERR KSPSetOperators(solver,
A,
A);
217 CHKERR KSPSetFromOptions(solver);
220 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
221 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
223 "TEST_PROBLEM",
COL,
D, INSERT_VALUES, SCATTER_REVERSE);
227 auto check_solution = [&] {
230 boost::shared_ptr<VectorDouble> field_vals_ptr(
new VectorDouble());
231 boost::shared_ptr<MatrixDouble> diff_field_vals_ptr(
new MatrixDouble());
233 fe.getOpPtrVector().push_back(
235 fe.getOpPtrVector().push_back(
237 fe.getOpPtrVector().push_back(
239 fe.getOpPtrVector().push_back(
241 fe.getOpPtrVector().push_back(
247 CHKERR assemble_matrices_and_vectors();
◆ approx_order
constexpr int approx_order = 6 |
|
staticconstexpr |
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
merge node from two bit levels
#define MYPCOMM_INDEX
default communicator number PCOMM
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
UBlasMatrix< double > MatrixDouble
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
auto createKSP(MPI_Comm comm)
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Calculate inverse of jacobian for face element.
Deprecated interface functions.
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
DeprecatedCoreInterface Interface
static constexpr int approx_order
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
Transform local reference derivatives of shape functions to global derivatives.
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
MoFEMErrorCode seedPrismsEntities(Range &prisms, const BitRefLevel &bit, int verb=-1)
Seed prism entities by bit level.
Get value at integration points for scalar field.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
Vector manager is used to create vectors \mofem_vectors.
Matrix manager is used to build and partition problems.
Operator for fat prism element updating integration weights in the volume.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode partitionSimpleProblem(const std::string name, int verb=VERBOSE)
partition problem dofs
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
UBlasVector< double > VectorDouble
const double D
diffusivity
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...