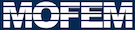 |
| v0.14.0
|
Go to the documentation of this file.
13 using namespace MoFEM;
15 namespace bio = boost::iostreams;
17 using bio::tee_device;
19 static char help[] =
"...\n\n";
21 int main(
int argc,
char *argv[]) {
23 const string default_options =
"-ksp_type fgmres \n"
25 "-pc_factor_mat_solver_type mumps \n"
26 "-mat_mumps_icntl_20 0 \n"
29 string param_file =
"param_file.petsc";
30 if (!
static_cast<bool>(ifstream(param_file))) {
31 std::ofstream file(param_file.c_str(), std::ios::ate);
33 file << default_options;
45 MPI_Comm_rank(PETSC_COMM_WORLD, &rank);
47 PetscBool flg = PETSC_TRUE;
51 if (flg != PETSC_TRUE) {
53 "*** ERROR -my_file (MESH FILE NEEDED)");
68 CHKERR moab.create_meshset(MESHSET_SET, meshset_level0);
93 if (flg != PETSC_TRUE) {
105 "MESH_NODE_POSITIONS");
121 "THERMAL_CONVECTION_FE");
138 "MESH_NODE_POSITIONS");
156 ->createMPIAIJWithArrays<PetscGlobalIdx_mi_tag>(
"THERMAL_PROBLEM", &
A);
161 true,
false,
false,
false);
163 true,
false,
false,
false);
174 CHKERR VecGhostUpdateBegin(T, INSERT_VALUES, SCATTER_FORWARD);
175 CHKERR VecGhostUpdateEnd(T, INSERT_VALUES, SCATTER_FORWARD);
177 CHKERR VecGhostUpdateBegin(
F, INSERT_VALUES, SCATTER_FORWARD);
178 CHKERR VecGhostUpdateEnd(
F, INSERT_VALUES, SCATTER_FORWARD);
185 "THERMAL_PROBLEM",
ROW, T, INSERT_VALUES, SCATTER_REVERSE);
188 thermal_elements.getLoopFeRhs());
190 thermal_elements.getLoopFeLhs());
194 "THERMAL_PROBLEM",
"THERMAL_CONVECTION_FE",
197 "THERMAL_PROBLEM",
"THERMAL_CONVECTION_FE",
204 CHKERR VecGhostUpdateBegin(
F, ADD_VALUES, SCATTER_REVERSE);
205 CHKERR VecGhostUpdateEnd(
F, ADD_VALUES, SCATTER_REVERSE);
208 CHKERR MatAssemblyBegin(
A, MAT_FINAL_ASSEMBLY);
209 CHKERR MatAssemblyEnd(
A, MAT_FINAL_ASSEMBLY);
215 CHKERR KSPCreate(PETSC_COMM_WORLD, &solver);
216 CHKERR KSPSetOperators(solver,
A,
A);
217 CHKERR KSPSetFromOptions(solver);
221 CHKERR VecGhostUpdateBegin(T, INSERT_VALUES, SCATTER_FORWARD);
222 CHKERR VecGhostUpdateEnd(T, INSERT_VALUES, SCATTER_FORWARD);
229 "THERMAL_PROBLEM",
ROW, T, INSERT_VALUES, SCATTER_REVERSE);
232 CHKERR moab.write_file(
"solution.h5m");
238 ent_method_on_10nodeTet.
setNodes =
false;
243 CHKERR moab.create_meshset(MESHSET_SET, out_meshset);
245 "THERMAL_PROBLEM",
"THERMAL_FE", out_meshset);
246 CHKERR moab.write_file(
"out.vtk",
"VTK",
"", &out_meshset, 1);
247 CHKERR moab.delete_entities(&out_meshset, 1);
253 CHKERR KSPDestroy(&solver);
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode problem_basic_method_postProcess(const Problem *problem_ptr, BasicMethod &method, int verb=DEFAULT_VERBOSITY)=0
Set data for BasicMethod.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
MoFEMErrorCode addHOOpsVol(const std::string field, E &e, bool h1, bool hcurl, bool hdiv, bool l2)
MoFEMErrorCode addThermalElements(const std::string field_name, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
add thermal element on tets
MoFEMErrorCode addThermalConvectionElement(const std::string field_name, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
add convection element
virtual int get_comm_rank() const =0
virtual MoFEMErrorCode get_problem_finite_elements_entities(const std::string name, const std::string &fe_name, const EntityHandle meshset)=0
add finite elements to the meshset
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
MyTriFE & getLoopFeFlux()
DeprecatedCoreInterface Interface
virtual MoFEMErrorCode problem_basic_method_preProcess(const Problem *problem_ptr, BasicMethod &method, int verb=DEFAULT_VERBOSITY)=0
Set data for BasicMethod.
MyTriFE & getLoopFeConvectionLhs()
#define CHKERR
Inline error check.
MoFEMErrorCode setThermalFluxFiniteElementRhsOperators(string field_name, Vec &F, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
this function is used in case of stationary problem for heat flux terms
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
MyTriFE & getLoopFeConvectionRhs()
MoFEMErrorCode addThermalFluxElement(const std::string field_name, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
add heat flux element
MoFEMErrorCode setThermalFiniteElementRhsOperators(string field_name, Vec &F)
this function is used in case of stationary problem to set elements for rhs
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
Vector manager is used to create vectors \mofem_vectors.
Matrix manager is used to build and partition problems.
structure grouping operators and data used for thermal problems
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode setThermalConvectionFiniteElementLhsOperators(string field_name, Mat A, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
const FTensor::Tensor2< T, Dim, Dim > Vec
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
MoFEMErrorCode setThermalConvectionFiniteElementRhsOperators(string field_name, Vec &F, const std::string mesh_nodals_positions="MESH_NODE_POSITIONS")
MoFEMErrorCode setThermalFiniteElementLhsOperators(string field_name, Mat A)
this function is used in case of stationary heat conductivity problem for lhs
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
int main(int argc, char *argv[])
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
MoFEMErrorCode partitionProblem(const std::string name, int verb=VERBOSE)
partition problem dofs (collective)