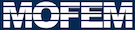 |
| v0.14.0
|
Go to the documentation of this file.
7 template <
class T,
int Tensor_Dim01,
int Tensor_Dim23>
8 class Ddg<T *, Tensor_Dim01, Tensor_Dim23>
14 mutable T *restrict data[(Tensor_Dim01 * (Tensor_Dim01 + 1)) / 2]
15 [(Tensor_Dim23 * (Tensor_Dim23 + 1)) / 2];
18 Ddg(T *d0000, T *d0010, T *d0011, T *d1000, T *d1010, T *d1011, T *d1100,
19 T *d1110, T *d1111,
const int i = 1)
22 ptr(0, 0, 0, 0) = d0000;
23 ptr(0, 0, 1, 0) = d0010;
24 ptr(0, 0, 1, 1) = d0011;
25 ptr(1, 0, 0, 0) = d1000;
26 ptr(1, 0, 1, 0) = d1010;
27 ptr(1, 0, 1, 1) = d1011;
28 ptr(1, 1, 0, 0) = d1100;
29 ptr(1, 1, 1, 0) = d1110;
30 ptr(1, 1, 1, 1) = d1111;
33 Ddg(T *d0000, T *d0001, T *d0002, T *d0011, T *d0012, T *d0022, T *d0100,
34 T *d0101, T *d0102, T *d0111, T *d0112, T *d0122, T *d0200, T *d0201,
35 T *d0202, T *d0211, T *d0212, T *d0222, T *d1100, T *d1101, T *d1102,
36 T *d1111, T *d1112, T *d1122, T *d1200, T *d1201, T *d1202, T *d1211,
37 T *d1212, T *d1222, T *d2200, T *d2201, T *d2202, T *d2211, T *d2212,
38 T *d2222,
const int i = 1)
41 ptr(0, 0, 0, 0) = d0000;
42 ptr(0, 0, 0, 1) = d0001;
43 ptr(0, 0, 0, 2) = d0002;
44 ptr(0, 0, 1, 1) = d0011;
45 ptr(0, 0, 1, 2) = d0012;
46 ptr(0, 0, 2, 2) = d0022;
47 ptr(0, 1, 0, 0) = d0100;
48 ptr(0, 1, 0, 1) = d0101;
49 ptr(0, 1, 0, 2) = d0102;
50 ptr(0, 1, 1, 1) = d0111;
51 ptr(0, 1, 1, 2) = d0112;
52 ptr(0, 1, 2, 2) = d0122;
53 ptr(0, 2, 0, 0) = d0200;
54 ptr(0, 2, 0, 1) = d0201;
55 ptr(0, 2, 0, 2) = d0202;
56 ptr(0, 2, 1, 1) = d0211;
57 ptr(0, 2, 1, 2) = d0212;
58 ptr(0, 2, 2, 2) = d0222;
59 ptr(1, 1, 0, 0) = d1100;
60 ptr(1, 1, 0, 1) = d1101;
61 ptr(1, 1, 0, 2) = d1102;
62 ptr(1, 1, 1, 1) = d1111;
63 ptr(1, 1, 1, 2) = d1112;
64 ptr(1, 1, 2, 2) = d1122;
65 ptr(1, 2, 0, 0) = d1200;
66 ptr(1, 2, 0, 1) = d1201;
67 ptr(1, 2, 0, 2) = d1202;
68 ptr(1, 2, 1, 1) = d1211;
69 ptr(1, 2, 1, 2) = d1212;
70 ptr(1, 2, 2, 2) = d1222;
71 ptr(2, 2, 0, 0) = d2200;
72 ptr(2, 2, 0, 1) = d2201;
73 ptr(2, 2, 0, 2) = d2202;
74 ptr(2, 2, 1, 1) = d2211;
75 ptr(2, 2, 1, 2) = d2212;
76 ptr(2, 2, 2, 2) = d2222;
80 template <
class...
U>
Ddg(
U *...
d) : data{
d...}, inc(1) {}
86 T &
operator()(
const int N1,
const int N2,
const int N3,
const int N4)
89 if(N1 >= Tensor_Dim01 || N1 < 0 || N2 >= Tensor_Dim01 || N2 < 0
90 || N3 >= Tensor_Dim23 || N3 < 0 || N4 >= Tensor_Dim23 || N4 < 0)
93 s <<
"Bad index in Dg<T*," << Tensor_Dim01 <<
"," << Tensor_Dim23
94 <<
">.operator(" << N1 <<
"," << N2 <<
"," << N3 <<
"," << N4
96 throw std::out_of_range(s.str());
100 ? (N3 > N4 ? *data[N1 + (N2 * (2 * Tensor_Dim01 - N2 - 1)) / 2]
101 [N3 + (N4 * (2 * Tensor_Dim23 - N4 - 1)) / 2]
102 : *data[N1 + (N2 * (2 * Tensor_Dim01 - N2 - 1)) / 2]
103 [N4 + (N3 * (2 * Tensor_Dim23 - N3 - 1)) / 2])
105 ? *data[N2 + (N1 * (2 * Tensor_Dim01 - N1 - 1)) / 2]
106 [N3 + (N4 * (2 * Tensor_Dim23 - N4 - 1)) / 2]
107 : *data[N2 + (N1 * (2 * Tensor_Dim01 - N1 - 1)) / 2]
108 [N4 + (N3 * (2 * Tensor_Dim23 - N3 - 1)) / 2]);
111 T
operator()(
const int N1,
const int N2,
const int N3,
const int N4)
const
114 if(N1 >= Tensor_Dim01 || N1 < 0 || N2 >= Tensor_Dim01 || N2 < 0
115 || N3 >= Tensor_Dim23 || N3 < 0 || N4 >= Tensor_Dim23 || N4 < 0)
118 s <<
"Bad index in Dg<T*," << Tensor_Dim01 <<
"," << Tensor_Dim23
119 <<
">.operator(" << N1 <<
"," << N2 <<
"," << N3 <<
"," << N4
120 <<
") const" << std::endl;
121 throw std::out_of_range(s.str());
125 ? (N3 > N4 ? *data[N1 + (N2 * (2 * Tensor_Dim01 - N2 - 1)) / 2]
126 [N3 + (N4 * (2 * Tensor_Dim23 - N4 - 1)) / 2]
127 : *data[N1 + (N2 * (2 * Tensor_Dim01 - N2 - 1)) / 2]
128 [N4 + (N3 * (2 * Tensor_Dim23 - N3 - 1)) / 2])
130 ? *data[N2 + (N1 * (2 * Tensor_Dim01 - N1 - 1)) / 2]
131 [N3 + (N4 * (2 * Tensor_Dim23 - N4 - 1)) / 2]
132 : *data[N2 + (N1 * (2 * Tensor_Dim01 - N1 - 1)) / 2]
133 [N4 + (N3 * (2 * Tensor_Dim23 - N3 - 1)) / 2]);
136 T *restrict &
ptr(
const int N1,
const int N2,
const int N3,
const int N4)
const
139 if(N1 >= Tensor_Dim01 || N1 < 0 || N2 >= Tensor_Dim01 || N2 < 0
140 || N3 >= Tensor_Dim23 || N3 < 0 || N4 >= Tensor_Dim23 || N4 < 0)
143 s <<
"Bad index in Dg<T," << Tensor_Dim01 <<
"," << Tensor_Dim23
144 <<
">.ptr(" << N1 <<
"," << N2 <<
"," << N3 <<
"," << N4 <<
")"
146 throw std::out_of_range(s.str());
150 ? (N3 > N4 ? data[N1 + (N2 * (2 * Tensor_Dim01 - N2 - 1)) / 2]
151 [N3 + (N4 * (2 * Tensor_Dim23 - N4 - 1)) / 2]
152 : data[N1 + (N2 * (2 * Tensor_Dim01 - N2 - 1)) / 2]
153 [N4 + (N3 * (2 * Tensor_Dim23 - N3 - 1)) / 2])
154 : (N3 > N4 ? data[N2 + (N1 * (2 * Tensor_Dim01 - N1 - 1)) / 2]
155 [N3 + (N4 * (2 * Tensor_Dim23 - N4 - 1)) / 2]
156 : data[N2 + (N1 * (2 * Tensor_Dim01 - N1 - 1)) / 2]
157 [N4 + (N3 * (2 * Tensor_Dim23 - N3 - 1)) / 2]);
165 template <
char i,
char j,
char k,
char l,
int Dim01,
int Dim23>
174 template <
char i,
char j,
char k,
char l,
int Dim01,
int Dim23>
182 Dim23,
i,
j,
k,
l>(*this);
188 template <
char i,
char j,
int N0,
int N1,
int Dim>
201 template <
char i,
char j,
int N0,
int N1,
int Dim>
216 template <
char i,
char j,
char k,
int N0,
int Dim1,
int Dim23>
218 Dim23, Dim1,
i,
j,
k>
228 template <
char i,
char j,
char k,
int N0,
int Dim1,
int Dim23>
230 Dim23, Dim1,
i,
j,
k>
243 template <
char i,
char j,
int Dim>
253 TensorExpr(*
this, N0, N1));
256 template <
char i,
char j,
int Dim>
260 const int N2,
const int N3)
const
265 TensorExpr(*
this, N2, N3));
270 template <
char i,
int Dim>
279 TensorExpr(*
this, N1, N2, N3));
282 template <
char i,
int Dim>
291 TensorExpr(*
this, N1, N2, N3));
298 template <
char i,
char j,
char k,
int Dim1,
int Dim23>
300 Dim23, Dim1,
i,
j,
k>
308 TensorExpr(*
this, N0));
315 for(
int i = 0;
i < (Tensor_Dim01 * (Tensor_Dim01 + 1)) / 2; ++
i)
316 for(
int j = 0;
j < (Tensor_Dim23 * (Tensor_Dim23 + 1)) / 2; ++
j)
326 template <
class T,
int Tensor_Dim01,
int Tensor_Dim23,
int I>
332 template <
class...
U>
333 Ddg(
U *...
d) :
Ddg<T *, Tensor_Dim01, Tensor_Dim23>(
d...) {}
339 for (
int i = 0;
i < (Tensor_Dim01 * (Tensor_Dim01 + 1)) / 2; ++
i)
340 for (
int j = 0;
j < (Tensor_Dim23 * (Tensor_Dim23 + 1)) / 2; ++
j)
Tensor2_symmetric_Expr< Ddg_numeral_01< const Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T >, T, Dim, i, j > operator()(const int N0, const int N1, const Index< i, Dim > index1, const Index< j, Dim > index2) const
Tensor2_symmetric_Expr< Ddg_number_rhs_01< Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T, N0, N1 >, T, Dim, i, j > operator()(const Number< N0 > n1, const Number< N1 > n2, const Index< i, Dim > index1, const Index< j, Dim > index2)
Tensor2_symmetric_Expr< Ddg_numeral_23< Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T >, T, Dim, i, j > operator()(const Index< i, Dim > index1, const Index< j, Dim > index2, const int N2, const int N3) const
Ddg(T *d0000, T *d0010, T *d0011, T *d1000, T *d1010, T *d1011, T *d1100, T *d1110, T *d1111, const int i=1)
const Tensor1_Expr< const dTensor0< T, Dim, i >, typename promote< T, double >::V, Dim, i > d(const Tensor0< T * > &a, const Index< i, Dim > index, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
Tensor2_symmetric_Expr< Ddg_number_01< const Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T, N0, N1 >, T, Dim, i, j > operator()(const Number< N0 > n1, const Number< N1 > n2, const Index< i, Dim > index1, const Index< j, Dim > index2) const
Ddg_Expr< Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T, Dim01, Dim23, i, j, k, l > operator()(const Index< i, Dim01 > index1, const Index< j, Dim01 > index2, const Index< k, Dim23 > index3, const Index< l, Dim23 > index4)
const Ddg & operator++() const
constexpr IntegrationType I
Dg_Expr< Ddg_number_rhs_0< Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T, N0 >, T, Dim23, Dim1, i, j, k > operator()(const Number< N0 > n1, const Index< k, Dim1 > index3, const Index< i, Dim23 > index1, const Index< j, Dim23 > index2)
T operator()(const int N1, const int N2, const int N3, const int N4) const
T *restrict & ptr(const int N1, const int N2, const int N3, const int N4) const
Dg_Expr< Ddg_number_0< const Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T, N0 >, T, Dim23, Dim1, i, j, k > operator()(const Number< N0 > n1, const Index< k, Dim1 > index3, const Index< i, Dim23 > index1, const Index< j, Dim23 > index2) const
FTensor::Index< 'i', SPACE_DIM > i
T & operator()(const int N1, const int N2, const int N3, const int N4)
Tensor1_Expr< Ddg_numeral_123< Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T >, T, Dim, i > operator()(const Index< i, Dim > index1, const int N1, const int N2, const int N3)
Tensor1_Expr< Ddg_numeral_123< Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T >, T, Dim, i > operator()(const int N1, const Index< i, Dim > index1, const int N2, const int N3)
FTensor::Index< 'j', 3 > j
Dg_Expr< Ddg_numeral_0< const Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T >, T, Dim23, Dim1, i, j, k > operator()(const int N0, const Index< k, Dim1 > index3, const Index< i, Dim23 > index1, const Index< j, Dim23 > index2) const
Ddg_Expr< const Ddg< T *, Tensor_Dim01, Tensor_Dim23 >, T, Dim01, Dim23, i, j, k, l > operator()(const Index< i, Dim01 > index1, const Index< j, Dim01 > index2, const Index< k, Dim23 > index3, const Index< l, Dim23 > index4) const
FTensor::Index< 'k', 3 > k
FTensor::Index< 'l', 3 > l
Ddg(T *d0000, T *d0001, T *d0002, T *d0011, T *d0012, T *d0022, T *d0100, T *d0101, T *d0102, T *d0111, T *d0112, T *d0122, T *d0200, T *d0201, T *d0202, T *d0211, T *d0212, T *d0222, T *d1100, T *d1101, T *d1102, T *d1111, T *d1112, T *d1122, T *d1200, T *d1201, T *d1202, T *d1211, T *d1212, T *d1222, T *d2200, T *d2201, T *d2202, T *d2211, T *d2212, T *d2222, const int i=1)
const Ddg & operator++() const