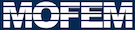 |
| v0.14.0
|
Go to the documentation of this file.
8 namespace bio = boost::iostreams;
13 static char help[] =
"...\n\n";
15 int main(
int argc,
char *argv[]) {
27 MPI_Comm_rank(PETSC_COMM_WORLD,&rank);
29 PetscBool flg = PETSC_TRUE;
31 #if PETSC_VERSION_GE(3,6,4)
36 if(flg != PETSC_TRUE) {
119 typedef tee_device<std::ostream, std::ofstream>
TeeDevice;
122 std::ofstream ofs(
"forces_and_sources_testing_ftensor.txt");
128 boost::shared_ptr<MatrixDouble> field1ValuesDataPtr;
129 boost::shared_ptr<VectorDouble> field2ValuesDataPtr;
130 boost::shared_ptr<MatrixDouble> grad1ValuesDataPtr;
131 boost::shared_ptr<MatrixDouble> grad2ValuesDataPtr;
135 boost::shared_ptr<MatrixDouble> field1_values_data_ptr,
136 boost::shared_ptr<VectorDouble> field2_values_data_ptr,
137 boost::shared_ptr<MatrixDouble> grad1_values_data_ptr,
138 boost::shared_ptr<MatrixDouble> grad2_values_data_ptr,
143 field1ValuesDataPtr(field1_values_data_ptr),
144 field2ValuesDataPtr(field2_values_data_ptr),
145 grad1ValuesDataPtr(grad1_values_data_ptr),
146 grad2ValuesDataPtr(grad2_values_data_ptr),
147 my_split(_my_split) {}
157 const int nb_gauss_pts = data.
getN().size1();
158 const int nb_base_functions = data.
getN().size2();
207 for(
int gg = 0;gg!=nb_gauss_pts;gg++) {
209 for(
int bb = 0;bb!=nb_base_functions;bb++) {
211 double base_val = base_function;
213 if(base_val!=data.
getN(gg)[bb]) {
218 for(
int dd = 0;
dd<3;
dd++) {
223 "Data inconsistency gg = %d bb = %d",
230 t2(
I,
J) = diff_base(
I)*diff_base(
J);
234 for(
int II = 0;II!=3;II++) {
235 for(
int JJ = 0;JJ!=3;JJ++) {
236 if(t2(II,JJ)-mat(II,JJ)!=0) {
257 int row_side,
int col_side,
258 EntityType row_type,EntityType col_type,
265 const int nb_gauss_pts = row_data.
getN().size1();
266 const int nb_base_functions_row = row_data.
getN().size2();
267 const int nb_base_functions_col = col_data.
getN().size2();
280 for(
int br = 0;br!=nb_base_functions_row;br++) {
284 for(
int bc = 0;bc!=nb_base_functions_col;bc++) {
288 auto field1_values = getFTensor1FromMat<3>(*field1ValuesDataPtr);
290 auto grad1_values = getFTensor2FromMat<3,3>(*grad1ValuesDataPtr);
291 auto grad2_values = getFTensor1FromMat<3>(*grad2ValuesDataPtr);
293 for(
int gg = 0;gg!=nb_gauss_pts;gg++) {
296 t2(
I,
J) = diff_base_row(
I)*diff_base_col(
J)*base_row*base_col;
297 t3(
I,
J,
K) = grad1_values(
I,
J)*field1_values(
K)*field2_values;
317 boost::shared_ptr<MatrixDouble> values1_at_gauss_pts_ptr =
319 boost::shared_ptr<VectorDouble> values2_at_gauss_pts_ptr =
321 boost::shared_ptr<MatrixDouble> grad1_at_gauss_pts_ptr =
323 boost::shared_ptr<MatrixDouble> grad2_at_gauss_pts_ptr =
333 "FIELD1", grad1_at_gauss_pts_ptr));
339 values1_at_gauss_pts_ptr,
340 values2_at_gauss_pts_ptr,
341 grad1_at_gauss_pts_ptr,
342 grad2_at_gauss_pts_ptr,
349 values1_at_gauss_pts_ptr,
350 values2_at_gauss_pts_ptr,
351 grad1_at_gauss_pts_ptr,
352 grad2_at_gauss_pts_ptr,
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Data on single entity (This is passed as argument to DataOperator::doWork)
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
Get values at integration pts for tensor filed rank 1, i.e. vector field.
UBlasMatrix< double > MatrixDouble
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
MatrixDouble & getDiffN(const FieldApproximationBase base)
get derivatives of base functions
FTensor::Index< 'J', DIM1 > J
@ OPROWCOL
operator doWork is executed on FE rows &columns
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
constexpr IntegrationType I
DeprecatedCoreInterface Interface
FTensor::Tensor0< FTensor::PackPtr< double *, 1 > > getFTensor0N(const FieldApproximationBase base)
Get base function as Tensor0.
static MoFEMErrorCodeGeneric< moab::ErrorCode > rval
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
int main(int argc, char *argv[])
static auto getFTensor0FromVec(ublas::vector< T, A > &data)
Get tensor rank 0 (scalar) form data vector.
Get value at integration points for scalar field.
friend class UserDataOperator
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
Volume finite element base.
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
const Tensor2_symmetric_Expr< const ddTensor0< T, Dim, i, j >, typename promote< T, double >::V, Dim, i, j > dd(const Tensor0< T * > &a, const Index< i, Dim > index1, const Index< j, Dim > index2, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
FTensor::Tensor1< FTensor::PackPtr< double *, Tensor_Dim >, Tensor_Dim > getFTensor1DiffN(const FieldApproximationBase base)
Get derivatives of base functions.
MatrixDouble & getN(const FieldApproximationBase base)
get base functions this return matrix (nb. of rows is equal to nb. of Gauss pts, nb....
MoFEMErrorCode partitionSimpleProblem(const std::string name, int verb=VERBOSE)
partition problem dofs
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
boost::ptr_deque< UserDataOperator > & getOpPtrVector()
Use to push back operator for row operator.
@ MOFEM_DATA_INCONSISTENCY
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
UBlasVector< double > VectorDouble
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
MatrixShallowArrayAdaptor< double > MatrixAdaptor
Matrix adaptor.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
@ OPROW
operator doWork function is executed on FE rows