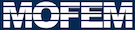 |
| v0.14.0
|
Go to the documentation of this file.
14 static char help[] =
"...\n\n";
36 return 200 * sin(x * 10.) * cos(y * 10.);
42 return sin(x * 10.) * cos(y * 10.);
64 :
domainField(
"U"), boundaryField(
"L"), mField(m_field) {}
98 auto get_ents_on_mesh = [&]() {
99 Range boundary_entities;
101 std::string entity_name = it->getName();
102 if (entity_name.compare(0, 18,
"BOUNDARY_CONDITION") == 0) {
104 boundary_entities,
true);
108 Range boundary_vertices;
110 boundary_vertices,
true);
111 boundary_entities.merge(boundary_vertices);
116 return boundary_entities;
119 auto mark_boundary_dofs = [&](
Range &&skin_edges) {
121 auto marker_ptr = boost::make_shared<std::vector<unsigned char>>();
123 skin_edges, *marker_ptr);
139 auto det_ptr = boost::make_shared<VectorDouble>();
140 auto jac_ptr = boost::make_shared<MatrixDouble>();
141 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
145 pipeline_mng->getOpDomainLhsPipeline().push_back(
147 pipeline_mng->getOpDomainLhsPipeline().push_back(
149 pipeline_mng->getOpDomainLhsPipeline().push_back(
151 pipeline_mng->getOpDomainLhsPipeline().push_back(
153 pipeline_mng->getOpDomainLhsPipeline().push_back(
159 pipeline_mng->getOpDomainRhsPipeline().push_back(
165 pipeline_mng->getOpBoundaryLhsPipeline().push_back(
167 pipeline_mng->getOpBoundaryLhsPipeline().push_back(
173 pipeline_mng->getOpBoundaryRhsPipeline().push_back(
185 auto domain_rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * (p - 1); };
186 auto domain_rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * (p - 1); };
187 CHKERR pipeline_mng->setDomainLhsIntegrationRule(domain_rule_lhs);
188 CHKERR pipeline_mng->setDomainRhsIntegrationRule(domain_rule_rhs);
190 auto boundary_rule_lhs = [](
int,
int,
int p) ->
int {
return 2 * p; };
191 auto boundary_rule_rhs = [](
int,
int,
int p) ->
int {
return 2 * p; };
192 CHKERR pipeline_mng->setBoundaryLhsIntegrationRule(boundary_rule_lhs);
193 CHKERR pipeline_mng->setBoundaryLhsIntegrationRule(boundary_rule_rhs);
203 auto ksp_solver = pipeline_mng->
createKSP();
204 CHKERR KSPSetFromOptions(ksp_solver);
214 CHKERR KSPGetPC(ksp_solver, &pc);
215 PetscBool is_pcfs = PETSC_FALSE;
216 PetscObjectTypeCompare((PetscObject)pc, PCFIELDSPLIT, &is_pcfs);
221 if (is_pcfs == PETSC_TRUE) {
222 IS is_domain, is_boundary;
223 cerr <<
"Running FIELDSPLIT..." << endl;
231 CHKERR PCFieldSplitSetIS(pc, NULL, is_boundary);
233 CHKERR ISDestroy(&is_boundary);
237 CHKERR KSPSetUp(ksp_solver);
243 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
244 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
255 pipeline_mng->getBoundaryLhsFE().reset();
257 auto d_ptr = boost::make_shared<VectorDouble>();
258 auto l_ptr = boost::make_shared<VectorDouble>();
262 auto post_proc_domain_fe = boost::make_shared<PostProcFaceEle>(
mField);
263 post_proc_domain_fe->getOpPtrVector().push_back(
265 post_proc_domain_fe->getOpPtrVector().push_back(
266 new OpPPMap(post_proc_domain_fe->getPostProcMesh(),
267 post_proc_domain_fe->getMapGaussPts(), {{domainField, d_ptr}},
269 pipeline_mng->getDomainRhsFE() = post_proc_domain_fe;
271 auto post_proc_boundary_fe = boost::make_shared<PostProcEdgeEle>(mField);
272 post_proc_boundary_fe->getOpPtrVector().push_back(
274 post_proc_boundary_fe->getOpPtrVector().push_back(
275 new OpPPMap(post_proc_boundary_fe->getPostProcMesh(),
276 post_proc_boundary_fe->getMapGaussPts(),
277 {{boundaryField, l_ptr}}, {}, {}, {}));
278 pipeline_mng->getBoundaryRhsFE() = post_proc_boundary_fe;
280 CHKERR pipeline_mng->loopFiniteElements();
281 CHKERR post_proc_domain_fe->writeFile(
"out_result_domain.h5m");
282 CHKERR post_proc_boundary_fe->writeFile(
"out_result_boundary.h5m");
301 int main(
int argc,
char *argv[]) {
304 const char param_file[] =
"param_file.petsc";
310 DMType dm_name =
"DMMOFEM";
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
static double sourceTermFunction(const double x, const double y, const double z)
Problem manager is used to build and partition problems.
MoFEMErrorCode assembleSystem()
std::string boundaryField
MoFEMErrorCode solveSystem()
int main(int argc, char *argv[])
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
constexpr auto domainField
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
PipelineManager interface.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
MoFEMErrorCode getDM(DM *dm)
Get DM.
Poisson2DLagrangeMultiplier(MoFEM::Interface &m_field)
#define CHKERR
Inline error check.
auto createDMVector(DM dm)
Get smart vector from DM.
virtual moab::Interface & get_moab()=0
MoFEMErrorCode readMesh()
MoFEMErrorCode outputResults()
implementation of Data Operators for Forces and Sources
MoFEMErrorCode setIntegrationRules()
Section manager is used to create indexes and sections.
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
Range boundaryEntitiesForFieldsplit
static double boundaryFunction(const double x, const double y, const double z)
MoFEMErrorCode boundaryCondition()
Get value at integration points for scalar field.
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
SmartPetscObj< KSP > createKSP(SmartPetscObj< DM > dm=nullptr)
Create KSP (linear) solver.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
Modify integration weights on face to take in account higher-order geometry.
MoFEMErrorCode markDofs(const std::string problem_name, RowColData rc, const enum MarkOP op, const Range ents, std::vector< unsigned char > &marker) const
Create vector with marked indices.
MoFEMErrorCode setupProblem()
@ AINSWORTH_BERNSTEIN_BEZIER_BASE
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
boost::shared_ptr< FEMethod > & getDomainLhsFE()
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
#define CATCH_ERRORS
Catch errors.
#define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet having a particular BC meshset in a moFEM field.
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
MoFEMErrorCode runProgram()
MoFEMErrorCode addBoundaryField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on boundary.
const double D
diffusivity
keeps basic data about problem
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
MoFEM::Interface & mField
const std::string getProblemName() const
Get the Problem Name.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
boost::shared_ptr< std::vector< unsigned char > > boundaryMarker
Post post-proc data at points from hash maps.