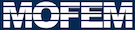 |
| v0.14.0
|
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
◆ Ele
◆ EntData
◆ OpEle
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
- Examples
- quad_polynomial_approximation.cpp.
Definition at line 83 of file quad_polynomial_approximation.cpp.
97 const char *list_bases[] = {
"ainsworth",
"ainsworth_lobatto",
"demkowicz",
100 PetscInt choice_base_value = AINSWORTH;
102 LASBASETOP, &choice_base_value, &flg);
104 if (flg != PETSC_TRUE)
107 if (choice_base_value == AINSWORTH)
109 if (choice_base_value == AINSWORTH_LOBATTO)
111 else if (choice_base_value == DEMKOWICZ)
113 else if (choice_base_value == BERNSTEIN)
116 enum spaces { H1SPACE, L2SPACE, LASBASETSPACE };
117 const char *list_spaces[] = {
"h1",
"l2"};
118 PetscInt choice_space_value = H1SPACE;
120 LASBASETSPACE, &choice_space_value, &flg);
121 if (flg != PETSC_TRUE)
124 if (choice_space_value == H1SPACE)
126 else if (choice_space_value == L2SPACE)
132 std::array<double, 12> one_quad_coords = {0, 0, 0,
140 std::array<EntityHandle, 4> one_quad_nodes;
141 for (
int n = 0;
n != 4; ++
n)
142 CHKERR moab.create_vertex(&one_quad_coords[3 *
n], one_quad_nodes[
n]);
144 CHKERR moab.create_element(MBQUAD, one_quad_nodes.data(), 4, one_quad);
145 Range one_quad_range;
146 one_quad_range.insert(one_quad);
147 Range one_quad_adj_ents;
148 CHKERR moab.get_adjacencies(one_quad_range, 1,
true, one_quad_adj_ents,
149 moab::Interface::UNION);
202 ->createMPIAIJWithArrays<PetscGlobalIdx_mi_tag>(
"TEST_PROBLEM",
A);
210 auto rule = [&](
int,
int,
int p) {
return 2 * (p + 1); };
212 auto assemble_matrices_and_vectors = [&]() {
215 fe.getRuleHook = rule;
216 auto jac_ptr = boost::make_shared<MatrixDouble>();
217 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
218 auto det_ptr = boost::make_shared<VectorDouble>();
220 fe.getOpPtrVector().push_back(
222 fe.getOpPtrVector().push_back(
224 fe.getOpPtrVector().push_back(
227 fe.getOpPtrVector().push_back(
new QuadOpRhs(
F));
228 fe.getOpPtrVector().push_back(
new QuadOpLhs(
A));
234 CHKERR MatAssemblyBegin(
A, MAT_FINAL_ASSEMBLY);
235 CHKERR MatAssemblyEnd(
A, MAT_FINAL_ASSEMBLY);
239 auto solve_problem = [&] {
241 auto solver =
createKSP(PETSC_COMM_WORLD);
242 CHKERR KSPSetOperators(solver,
A,
A);
243 CHKERR KSPSetFromOptions(solver);
246 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
247 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
249 "TEST_PROBLEM",
COL,
D, INSERT_VALUES, SCATTER_REVERSE);
253 auto check_solution = [&] {
256 fe.getRuleHook = rule;
257 auto field_vals_ptr = boost::make_shared<VectorDouble>();
258 auto diff_field_vals_ptr = boost::make_shared<MatrixDouble>();
259 auto jac_ptr = boost::make_shared<MatrixDouble>();
260 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
261 auto det_ptr = boost::make_shared<VectorDouble>();
263 fe.getOpPtrVector().push_back(
266 fe.getOpPtrVector().push_back(
268 fe.getOpPtrVector().push_back(
270 fe.getOpPtrVector().push_back(
274 "FIELD1", diff_field_vals_ptr, space ==
L2 ? MBQUAD : MBVERTEX));
275 fe.getOpPtrVector().push_back(
276 new QuadOpCheck(field_vals_ptr, diff_field_vals_ptr));
281 CHKERR assemble_matrices_and_vectors();
◆ approx_order
constexpr int approx_order = 6 |
|
staticconstexpr |
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
@ L2
field with C-1 continuity
static constexpr int approx_order
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
auto createKSP(MPI_Comm comm)
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
DeprecatedCoreInterface Interface
FieldSpace
approximation spaces
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
Get value at integration points for scalar field.
Modify integration weights on face to take in account higher-order geometry.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
Vector manager is used to create vectors \mofem_vectors.
@ AINSWORTH_BERNSTEIN_BEZIER_BASE
Matrix manager is used to build and partition problems.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode partitionSimpleProblem(const std::string name, int verb=VERBOSE)
partition problem dofs
MoFEM::PipelineManager::ElementsAndOpsByDim< SPACE_DIM >::DomainEle Ele
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
FieldApproximationBase
approximation base
const double D
diffusivity
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...