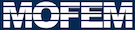 |
| v0.14.0
|
Go to the documentation of this file.
12 using namespace MoFEM;
14 static char help[] =
"...\n\n";
41 double operator()(
const double x,
const double y,
const double z) {
42 return sin(x * 10.) * cos(y * 10.);
78 boost::shared_ptr<CommonData> commonDataPtr;
88 OpError(boost::shared_ptr<CommonData> &common_data_ptr)
93 if (
const size_t nb_dofs = data.
getIndices().size()) {
95 const int nb_integration_pts = getGaussPts().size2();
96 auto t_w = getFTensor0IntegrationWeight();
98 auto t_coords = getFTensor1CoordsAtGaussPts();
104 const double volume = getMeasure();
108 for (
int gg = 0; gg != nb_integration_pts; ++gg) {
110 const double alpha = t_w * volume;
113 error += alpha * pow(diff, 2);
115 for (
size_t r = 0;
r != nb_dofs; ++
r) {
116 nf[
r] += alpha * t_row_base * diff;
126 CHKERR VecSetValue(commonDataPtr->L2Vec, index, error, ADD_VALUES);
139 CHKERR setIntegrationRules();
140 CHKERR createCommonData();
141 CHKERR boundaryCondition();
154 CHKERR mField.getInterface(simpleInterface);
155 CHKERR simpleInterface->getOptions();
156 CHKERR simpleInterface->loadFile();
168 constexpr
int order = 4;
170 CHKERR simpleInterface->setUp();
179 auto rule = [](
int,
int,
int p) ->
int {
return 2 * p; };
192 commonDataPtr = boost::make_shared<CommonData>();
195 mField.get_comm(), (!mField.get_comm_rank()) ? 1 : 0, 1);
196 commonDataPtr->approxVals = boost::make_shared<VectorDouble>();
223 CHKERR KSPSetFromOptions(solver);
226 auto dm = simpleInterface->getDM();
231 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
232 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
242 auto post_proc_fe = boost::make_shared<PostProcEle>(mField);
244 auto u_ptr = boost::make_shared<VectorDouble>();
245 post_proc_fe->getOpPtrVector().push_back(
250 post_proc_fe->getOpPtrVector().push_back(
254 post_proc_fe->getPostProcMesh(), post_proc_fe->getMapGaussPts(),
256 {{FIELD_NAME, u_ptr}},
268 CHKERR post_proc_fe->writeFile(
"out_approx.h5m");
294 PetscPrintf(PETSC_COMM_SELF,
"Error %6.4e Vec norm %6.4e\n", std::sqrt(array[0]),
297 constexpr
double eps = 1e-8;
300 "Not converged solution");
305 int main(
int argc,
char *argv[]) {
308 const char param_file[] =
"param_file.petsc";
314 DMType dm_name =
"DMMOFEM";
int main(int argc, char *argv[])
[Check results]
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
static ApproxFieldFunction< FIELD_DIM > approxFunction
Data on single entity (This is passed as argument to DataOperator::doWork)
MoFEMErrorCode checkResults()
[Postprocess results]
boost::shared_ptr< FEMethod > & getDomainRhsFE()
MoFEMErrorCode assembleSystem()
[Push operators to pipeline]
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, FIELD_DIM > OpDomainSource
Example(MoFEM::Interface &m_field)
SmartPetscObj< Vec > L2Vec
MoFEMErrorCode loopFiniteElements(SmartPetscObj< DM > dm=nullptr)
Iterate finite elements.
double operator()(const double x, const double y, const double z)
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
boost::ptr_deque< UserDataOperator > & getOpDomainRhsPipeline()
Get the Op Domain Rhs Pipeline object.
MoFEMErrorCode doWork(int side, EntityType type, EntData &data)
virtual int get_comm_rank() const =0
boost::shared_ptr< VectorDouble > approxVals
PipelineManager interface.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, FIELD_DIM > OpDomainMass
MoFEMErrorCode VecSetValues(Vec V, const EntitiesFieldData::EntData &data, const double *ptr, InsertMode iora)
Assemble PETSc vector.
Deprecated interface functions.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, FIELD_DIM > OpDomainMass
DeprecatedCoreInterface Interface
FTensor::Tensor0< FTensor::PackPtr< double *, 1 > > getFTensor0N(const FieldApproximationBase base)
Get base function as Tensor0.
MoFEMErrorCode readMesh()
[Run problem]
#define CHKERR
Inline error check.
auto createDMVector(DM dm)
Get smart vector from DM.
implementation of Data Operators for Forces and Sources
MoFEM::FaceElementForcesAndSourcesCore FaceEle
OpError(boost::shared_ptr< CommonData > &common_data_ptr)
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
static auto getFTensor0FromVec(ublas::vector< T, A > &data)
Get tensor rank 0 (scalar) form data vector.
MoFEMErrorCode solveSystem()
[Solve]
Get value at integration points for scalar field.
SmartPetscObj< KSP > createKSP(SmartPetscObj< DM > dm=nullptr)
Create KSP (linear) solver.
const VectorInt & getIndices() const
Get global indices of dofs on entity.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
MoFEMErrorCode setIntegrationRules()
[Set up problem]
MoFEMErrorCode setDomainRhsIntegrationRule(RuleHookFun rule)
boost::shared_ptr< CommonData > commonDataPtr
boost::ptr_deque< UserDataOperator > & getOpDomainLhsPipeline()
Get the Op Domain Lhs Pipeline object.
Volume finite element base.
FTensor::Index< 'i', SPACE_DIM > i
MoFEMErrorCode setDomainLhsIntegrationRule(RuleHookFun rule)
boost::shared_ptr< FEMethod > & getDomainLhsFE()
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
constexpr char FIELD_NAME[]
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode setupProblem()
[Run problem]
ForcesAndSourcesCore::UserDataOperator UserDataOperator
MoFEMErrorCode boundaryCondition()
[Set up problem]
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
@ MOFEM_DATA_INCONSISTENCY
EntitiesFieldData::EntData EntData
auto createVectorMPI(MPI_Comm comm, PetscInt n, PetscInt N)
Create MPI Vector.
MoFEMErrorCode runProblem()
[Run problem]
UBlasVector< double > VectorDouble
const double D
diffusivity
MoFEM::Interface & mField
MoFEMErrorCode createCommonData()
[Set up problem]
ElementsAndOps< SPACE_DIM >::DomainEle DomainEle
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, FIELD_DIM > OpDomainSource
MoFEMErrorCode outputResults()
[Solve]
boost::shared_ptr< CommonData > commonDataPtr
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
SmartPetscObj< Vec > resVec
Post post-proc data at points from hash maps.