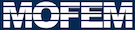 |
| v0.14.0
|
Mix transport problem.
More...
#include <tutorials/cor-0to1/src/MixTransportElement.hpp>
|
| MixTransportElement (MoFEM::Interface &m_field) |
| construction of this data structure More...
|
|
virtual | ~MixTransportElement () |
| destructor More...
|
|
MoFEMErrorCode | getDirichletBCIndices (IS *is) |
| get dof indices where essential boundary conditions are applied More...
|
|
virtual MoFEMErrorCode | getSource (const EntityHandle ent, const double x, const double y, const double z, double &flux) |
| set source term More...
|
|
virtual MoFEMErrorCode | getResistivity (const EntityHandle ent, const double x, const double y, const double z, MatrixDouble3by3 &inv_k) |
| natural (Dirichlet) boundary conditions (set values) More...
|
|
virtual MoFEMErrorCode | getBcOnValues (const EntityHandle ent, const int gg, const double x, const double y, const double z, double &value) |
| evaluate natural (Dirichlet) boundary conditions More...
|
|
virtual MoFEMErrorCode | getBcOnFluxes (const EntityHandle ent, const double x, const double y, const double z, double &flux) |
| essential (Neumann) boundary condition (set fluxes) More...
|
|
MoFEMErrorCode | addFields (const std::string &values, const std::string &fluxes, const int order) |
| Add fields to database. More...
|
|
MoFEMErrorCode | addFiniteElements (const std::string &fluxes_name, const std::string &values_name) |
| add finite elements More...
|
|
MoFEMErrorCode | buildProblem (BitRefLevel &ref_level) |
| Build problem. More...
|
|
MoFEMErrorCode | postProc (const string out_file) |
| Post process results. More...
|
|
MoFEMErrorCode | createMatrices () |
| create matrices More...
|
|
MoFEMErrorCode | solveLinearProblem () |
| solve problem More...
|
|
MoFEMErrorCode | calculateResidual () |
| calculate residual More...
|
|
MoFEMErrorCode | evaluateError () |
| Calculate error on elements. More...
|
|
MoFEMErrorCode | destroyMatrices () |
| destroy matrices More...
|
|
Mix transport problem.
Note to solve this system you need to use direct solver or proper preconditioner for saddle problem.
It is based on [6] [7] [reviartthomas1996] https://www.researchgate.net/profile/Richard_Falk/publication/226454406_Differential_Complexes_and_Stability_of_Finite_Element_Methods_I._The_de_Rham_Complex/links/02e7e5214f0426ff77000000.pdf
General problem have form,
\[ \mathbf{A} \boldsymbol\sigma + \textrm{grad}[u] = \mathbf{0} \; \textrm{on} \; \Omega \\ \textrm{div}[\boldsymbol\sigma] = f \; \textrm{on} \; \Omega \]
Definition at line 34 of file MixTransportElement.hpp.
◆ MixTransportElement()
MixTransport::MixTransportElement::MixTransportElement |
( |
MoFEM::Interface & |
m_field | ) |
|
|
inline |
◆ ~MixTransportElement()
virtual MixTransport::MixTransportElement::~MixTransportElement |
( |
| ) |
|
|
inlinevirtual |
◆ addFields()
MoFEMErrorCode MixTransport::MixTransportElement::addFields |
( |
const std::string & |
values, |
|
|
const std::string & |
fluxes, |
|
|
const int |
order |
|
) |
| |
|
inline |
Add fields to database.
- Parameters
-
values | name of the fields |
fluxes | name of filed for fluxes |
order | order of approximation |
- Returns
- error code
Definition at line 194 of file MixTransportElement.hpp.
◆ addFiniteElements()
MoFEMErrorCode MixTransport::MixTransportElement::addFiniteElements |
( |
const std::string & |
fluxes_name, |
|
|
const std::string & |
values_name |
|
) |
| |
|
inline |
add finite elements
Definition at line 213 of file MixTransportElement.hpp.
250 CHKERR it->getAttributeDataStructure(temp_data);
252 temp_data.
data.Conductivity;
253 setOfBlocks[it->getMeshsetId()].cApacity = temp_data.
data.HeatCapacity;
255 it->meshset, MBTET,
setOfBlocks[it->getMeshsetId()].tEts,
true);
257 setOfBlocks[it->getMeshsetId()].tEts, MBTET,
"MIX");
261 setOfBlocks[it->getMeshsetId()].tEts, 2,
false, skeleton,
262 moab::Interface::UNION);
◆ buildProblem()
Build problem.
- Parameters
-
ref_level | mesh refinement on which mesh problem you like to built. |
- Returns
- error code
Definition at line 296 of file MixTransportElement.hpp.
312 ref_tets = subtract(ref_tets, done_tets);
326 ref_faces = subtract(ref_faces, done_faces);
◆ calculateResidual()
MoFEMErrorCode MixTransport::MixTransportElement::calculateResidual |
( |
| ) |
|
|
inline |
calculate residual
Definition at line 617 of file MixTransportElement.hpp.
620 CHKERR VecGhostUpdateBegin(
F, INSERT_VALUES, SCATTER_FORWARD);
621 CHKERR VecGhostUpdateEnd(
F, INSERT_VALUES, SCATTER_FORWARD);
629 new OpFluxDivergenceAtGaussPts(*
this,
"FLUXES"));
632 new OpDivTauU_HdivL2(*
this,
"FLUXES",
"VALUES",
F));
634 new OpTauDotSigma_HdivHdiv(*
this,
"FLUXES", PETSC_NULL,
F));
636 new OpVDivSigma_L2Hdiv(*
this,
"VALUES",
"FLUXES", PETSC_NULL,
F));
641 CHKERR VecGhostUpdateBegin(
F, ADD_VALUES, SCATTER_REVERSE);
642 CHKERR VecGhostUpdateEnd(
F, ADD_VALUES, SCATTER_REVERSE);
648 std::vector<int> ids;
650 std::vector<double> vals(ids.size(), 0);
658 CHKERR VecNorm(
F, NORM_2, &nrm2_F);
659 PetscPrintf(PETSC_COMM_WORLD,
"nrm2_F = %6.4e\n", nrm2_F);
660 const double eps = 1e-8;
◆ createMatrices()
◆ destroyMatrices()
◆ evaluateError()
Calculate error on elements.
- Todo:
- this functions runs serial, in future should be parallel and work on distributed meshes.
Definition at line 675 of file MixTransportElement.hpp.
688 new OpFluxDivergenceAtGaussPts(*
this,
"FLUXES"));
690 new OpValuesGradientAtGaussPts(*
this,
"VALUES"));
697 "Nb dofs %d error flux^2 = %6.4e error div^2 = %6.4e error "
698 "jump^2 = %6.4e error tot^2 = %6.4e\n",
◆ getBcOnFluxes()
◆ getBcOnValues()
◆ getDirichletBCIndices()
MoFEMErrorCode MixTransport::MixTransportElement::getDirichletBCIndices |
( |
IS * |
is | ) |
|
|
inline |
get dof indices where essential boundary conditions are applied
- Parameters
-
- Returns
- error code
Definition at line 92 of file MixTransportElement.hpp.
98 ids.empty() ? PETSC_NULL : &ids[0],
99 PETSC_COPY_VALUES, &is_local);
100 CHKERR ISAllGather(is_local, is);
101 CHKERR ISDestroy(&is_local);
◆ getResistivity()
natural (Dirichlet) boundary conditions (set values)
- Parameters
-
ent | handle to finite element entity |
x | coord |
y | coord |
z | coord |
value | reference to value set by function |
- Returns
- error code
Definition at line 131 of file MixTransportElement.hpp.
136 for (
int dd = 0;
dd < 3;
dd++) {
◆ getSource()
set source term
- Parameters
-
ent | handle to entity on which function is evaluated |
x | coord |
y | coord |
z | coord |
flux | reference to source term set by function |
- Returns
- error code
Reimplemented in MyTransport, and MyTransport.
Definition at line 114 of file MixTransportElement.hpp.
◆ postProc()
MoFEMErrorCode MixTransport::MixTransportElement::postProc |
( |
const string |
out_file | ) |
|
|
inline |
Post process results.
- Returns
- error code
Definition at line 433 of file MixTransportElement.hpp.
441 auto values_ptr = boost::make_shared<VectorDouble>();
442 auto grad_ptr = boost::make_shared<MatrixDouble>();
443 auto flux_ptr = boost::make_shared<MatrixDouble>();
445 post_proc.getOpPtrVector().push_back(
447 post_proc.getOpPtrVector().push_back(
449 post_proc.getOpPtrVector().push_back(
454 post_proc.getOpPtrVector().push_back(
456 new OpPPMap(post_proc.getPostProcMesh(), post_proc.getMapGaussPts(),
458 {{
"VALUES", values_ptr}},
460 {{
"GRAD", grad_ptr}, {
"FLUXES", flux_ptr}},
466 post_proc.getOpPtrVector().push_back(
new OpPostProc(
467 post_proc.getPostProcMesh(), post_proc.getMapGaussPts()));
471 CHKERR post_proc.writeFile(out_file.c_str());
◆ solveLinearProblem()
MoFEMErrorCode MixTransport::MixTransportElement::solveLinearProblem |
( |
| ) |
|
|
inline |
solve problem
- Returns
- error code
Definition at line 493 of file MixTransportElement.hpp.
497 CHKERR VecGhostUpdateBegin(
F, INSERT_VALUES, SCATTER_FORWARD);
498 CHKERR VecGhostUpdateEnd(
F, INSERT_VALUES, SCATTER_FORWARD);
500 CHKERR VecGhostUpdateBegin(
D0, INSERT_VALUES, SCATTER_FORWARD);
501 CHKERR VecGhostUpdateEnd(
D0, INSERT_VALUES, SCATTER_FORWARD);
503 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
504 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
507 "MIX",
COL,
D, INSERT_VALUES, SCATTER_REVERSE);
520 CHKERR VecGhostUpdateBegin(
D0, INSERT_VALUES, SCATTER_REVERSE);
521 CHKERR VecGhostUpdateEnd(
D0, INSERT_VALUES, SCATTER_REVERSE);
530 new OpFluxDivergenceAtGaussPts(*
this,
"FLUXES"));
533 new OpDivTauU_HdivL2(*
this,
"FLUXES",
"VALUES",
F));
535 new OpTauDotSigma_HdivHdiv(*
this,
"FLUXES",
Aij,
F));
537 new OpVDivSigma_L2Hdiv(*
this,
"VALUES",
"FLUXES",
Aij,
F));
547 CHKERR MatAssemblyBegin(
Aij, MAT_FINAL_ASSEMBLY);
548 CHKERR MatAssemblyEnd(
Aij, MAT_FINAL_ASSEMBLY);
549 CHKERR VecGhostUpdateBegin(
F, ADD_VALUES, SCATTER_REVERSE);
550 CHKERR VecGhostUpdateEnd(
F, ADD_VALUES, SCATTER_REVERSE);
556 CHKERR VecNorm(
F, NORM_2, &nrm2_F);
557 PetscPrintf(PETSC_COMM_WORLD,
"nrm2_F = %6.4e\n", nrm2_F);
568 CHKERR MatZeroRowsColumnsIS(
Aij, essential_bc_ids, 1,
D0,
F);
569 CHKERR ISDestroy(&essential_bc_ids);
579 CHKERR VecNorm(
F, NORM_2, &nrm2_F);
580 PetscPrintf(PETSC_COMM_WORLD,
"With BC nrm2_F = %6.4e\n", nrm2_F);
593 CHKERR KSPCreate(PETSC_COMM_WORLD, &solver);
595 CHKERR KSPSetFromOptions(solver);
598 CHKERR KSPDestroy(&solver);
602 CHKERR VecNorm(
D, NORM_2, &nrm2_D);
604 PetscPrintf(PETSC_COMM_WORLD,
"nrm2_D = %6.4e\n", nrm2_D);
606 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
607 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
611 "MIX",
COL,
D, INSERT_VALUES, SCATTER_REVERSE);
◆ Aij
Mat MixTransport::MixTransportElement::Aij |
◆ bcIndices
set<int> MixTransport::MixTransportElement::bcIndices |
Vec MixTransport::MixTransportElement::D |
◆ D0
Vec MixTransport::MixTransportElement::D0 |
◆ divergenceAtGaussPts
VectorDouble MixTransport::MixTransportElement::divergenceAtGaussPts |
◆ errorMap
Vec MixTransport::MixTransportElement::F |
◆ feTri
MyTriFE MixTransport::MixTransportElement::feTri |
◆ feVol
MyVolumeFE MixTransport::MixTransportElement::feVol |
◆ fluxesAtGaussPts
MatrixDouble MixTransport::MixTransportElement::fluxesAtGaussPts |
◆ mField
◆ setOfBlocks
std::map<int, BlockData> MixTransport::MixTransportElement::setOfBlocks |
◆ sumErrorDiv
double MixTransport::MixTransportElement::sumErrorDiv |
◆ sumErrorFlux
double MixTransport::MixTransportElement::sumErrorFlux |
◆ sumErrorJump
double MixTransport::MixTransportElement::sumErrorJump |
◆ valuesAtGaussPts
VectorDouble MixTransport::MixTransportElement::valuesAtGaussPts |
◆ valuesGradientAtGaussPts
MatrixDouble MixTransport::MixTransportElement::valuesGradientAtGaussPts |
The documentation for this struct was generated from the following file:
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
virtual MoFEMErrorCode modify_problem_ref_level_set_bit(const std::string &name_problem, const BitRefLevel &bit)=0
set ref level for problem
Problem manager is used to build and partition problems.
virtual MPI_Comm & get_comm() const =0
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
@ L2
field with C-1 continuity
#define _IT_CUBITMESHSETS_BY_BCDATA_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet in a moFEM field.
virtual const Problem * get_problem(const std::string problem_name) const =0
Get the problem object.
MoFEMErrorCode getDirichletBCIndices(IS *is)
get dof indices where essential boundary conditions are applied
MoFEMErrorCode VecSetValues(Vec V, const EntitiesFieldData::EntData &data, const double *ptr, InsertMode iora)
Assemble PETSc vector.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
@ MAT_THERMALSET
block name is "MAT_THERMAL"
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
Get vector field for H-div approximation.
DofIdx getNbDofsRow() const
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
Get value at integration points for scalar field.
MyTriFE feTri
Instance of surface element.
virtual int get_comm_size() const =0
std::map< int, BlockData > setOfBlocks
maps block set id with appropriate BlockData
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
Vector manager is used to create vectors \mofem_vectors.
MyVolumeFE feVol
Instance of volume element.
Matrix manager is used to build and partition problems.
Add operators pushing bases from local to physical configuration.
const Tensor2_symmetric_Expr< const ddTensor0< T, Dim, i, j >, typename promote< T, double >::V, Dim, i, j > dd(const Tensor0< T * > &a, const Index< i, Dim > index1, const Index< j, Dim > index2, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
Thermal material data structure.
#define BITREFLEVEL_SIZE
max number of refinements
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
boost::ptr_deque< UserDataOperator > & getOpPtrVector()
Use to push back operator for row operator.
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
map< double, EntityHandle > errorMap
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
keeps basic data about problem
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
MoFEM::Interface & mField
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
@ HDIV
field with continuous normal traction
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode partitionProblem(const std::string name, int verb=VERBOSE)
partition problem dofs (collective)
Post post-proc data at points from hash maps.