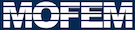 |
| v0.14.0
|
Go to the documentation of this file.
14 using namespace MoFEM;
16 static char help[] =
"...\n\n";
72 :
OpBase(
"T",
"T",
OpBase::OPROWCOL), approxVals(approx_vals) {
86 :
OpBase(
"T",
"T",
OpBase::OPROW), approxVals(approx_vals) {}
111 boost::shared_ptr<VectorDouble> &approx_vals,
double &sum_temp,
114 sumTemperature(sum_temp), surfaceArea(surf) {}
124 CHKERR createCommonData();
140 constexpr
int order = 3;
150 approxVals = boost::make_shared<VectorDouble>();
151 approxGradVals = boost::make_shared<MatrixDouble>();
160 auto set_initial_temperature = [&](
VectorAdaptor &&field_data,
double *xcoord,
161 double *ycoord,
double *zcoord) {
167 CHKERR mField.getInterface(field_blas);
181 auto det_ptr = boost::make_shared<VectorDouble>();
182 auto jac_ptr = boost::make_shared<MatrixDouble>();
183 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
216 new OpRadiationRhs(approxVals));
223 new OpRadiationLhs(approxVals));
237 CHKERR TSSetDuration(ts, PETSC_DEFAULT, ftime);
238 CHKERR TSSetFromOptions(ts);
239 CHKERR TSSetExactFinalTime(ts, TS_EXACTFINALTIME_MATCHSTEP);
246 CHKERR TSGetTime(ts, &ftime);
248 PetscInt steps, snesfails, rejects, nonlinits, linits;
249 CHKERR TSGetTimeStepNumber(ts, &steps);
250 CHKERR TSGetSNESFailures(ts, &snesfails);
251 CHKERR TSGetStepRejections(ts, &rejects);
252 CHKERR TSGetSNESIterations(ts, &nonlinits);
253 CHKERR TSGetKSPIterations(ts, &linits);
255 "steps %d (%d rejected, %d SNES fails), ftime %g, nonlinits "
257 steps, rejects, snesfails, ftime, nonlinits, linits);
271 boost::make_shared<PostProcBrokenMeshInMoab<DomainEle>>(mField);
273 auto t_ptr = boost::make_shared<VectorDouble>();
274 post_proc_fe->getOpPtrVector().push_back(
279 post_proc_fe->getOpPtrVector().push_back(
281 new OpPPMap(post_proc_fe->getPostProcMesh(),
282 post_proc_fe->getMapGaussPts(),
297 double sum_temperature;
300 new OpCalcSurfaceAverageTemperature(approxVals, sum_temperature,
307 CHKERR post_proc_fe->writeFile(
"out_radiation.h5m");
309 MOFEM_LOG_C(
"EXAMPLE", Sev::inform,
"Surface area %3.4e [km]", surface_area);
311 "Average subsurface temperatute %3.4e [K]",
312 sum_temperature / surface_area);
322 int main(
int argc,
char *argv[]) {
325 const char param_file[] =
"param_file.petsc";
329 auto core_log = logging::core::get();
331 LogManager::createSink(LogManager::getStrmWorld(),
"EXAMPLE"));
332 LogManager::setLog(
"EXAMPLE");
338 DMType dm_name =
"DMMOFEM";
373 const double vol = this->getMeasure();
375 auto t_w = getFTensor0IntegrationWeight();
381 auto t_coords = getFTensor1CoordsAtGaussPts();
387 const double r_cylinder = t_coords(0);
390 const double alpha = t_w * vol *
Beta * (2 * M_PI * r_cylinder);
392 for (
int rr = 0; rr !=
nbRows; ++rr) {
395 for (
int cc = 0; cc !=
nbCols; cc++) {
396 if (std::abs(t_coords(0)) > std::numeric_limits<double>::epsilon()) {
397 locMat(rr, cc) += alpha * t_row_base * t_col_base * 4 * pow(t_val, 3);
416 const double vol = getMeasure();
418 auto t_w = getFTensor0IntegrationWeight();
424 auto t_coords = getFTensor1CoordsAtGaussPts();
427 for (
int gg = 0; gg != nbIntegrationPts; gg++) {
430 const double r_cylinder = t_coords(0);
433 const double alpha = t_w * vol *
Beta * (2 * M_PI * r_cylinder);
435 for (
int rr = 0; rr != nbRows; ++rr) {
436 if (std::abs(t_coords(0)) > std::numeric_limits<double>::epsilon()) {
437 locF[rr] += alpha * t_row_base * (pow(t_val, 4) - pow(
T_ambient, 4));
454 const double vol = getMeasure();
456 auto t_w = getFTensor0IntegrationWeight();
460 auto t_coords = getFTensor1CoordsAtGaussPts();
462 const double time = getFEMethod()->ts_t;
465 constexpr
double flux_p = -0.03e6;
466 constexpr
double flux_c = -0.23e6;
469 for (
int gg = 0; gg != nbIntegrationPts; gg++) {
472 const double r_cylinder = t_coords(0);
474 const double r = std::sqrt(t_coords(
i) * t_coords(
i));
475 const double s = std::abs(t_coords(1)) /
r;
478 const double alpha = t_w * vol * (2 * M_PI * r_cylinder);
480 for (
int rr = 0; rr != nbRows; ++rr) {
481 locF[rr] += alpha * t_row_base * (s * flux_p + flux_c) * time;
497 if (
type == MBVERTEX) {
499 const double vol = getMeasure();
501 auto t_w = getFTensor0IntegrationWeight();
505 auto t_coords = getFTensor1CoordsAtGaussPts();
507 size_t nb_integration_pts = getGaussPts().size2();
510 for (
auto gg = 0; gg != nb_integration_pts; ++gg) {
513 const double r_cylinder = t_coords(0);
516 const double alpha = t_w * vol * (2 * M_PI * r_cylinder);
518 sumTemperature += alpha * t_val;
519 surfaceArea += alpha;
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
int nbIntegrationPts
number of integration points
Data on single entity (This is passed as argument to DataOperator::doWork)
MoFEMErrorCode checkResults()
[Postprocess results]
boost::shared_ptr< FEMethod > & getDomainRhsFE()
boost::shared_ptr< VectorDouble > approxVals
MoFEMErrorCode setVertexDofs(VertexCoordsFunction lambda, const std::string field_name, Range *verts=nullptr)
Set DOFs on vertices using user function.
MoFEMErrorCode OPs()
[Boundary condition]
static int integrationRule(int, int, int p_data)
MatrixDouble locMat
local entity block matrix
Example(MoFEM::Interface &m_field)
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpGradTimesTensor< 1, 1, SPACE_DIM > OpDomainGradTimesVec
FTensor::Index< 'i', 2 > i
summit Index
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpGradTimesTensor< 1, 1, 2 > OpDomainGradTimesVec
MoFEMErrorCode loopFiniteElements(SmartPetscObj< DM > dm=nullptr)
Iterate finite elements.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode kspSolve()
[Push operators to pipeline]
boost::shared_ptr< FEMethod > & getBoundaryLhsFE()
boost::shared_ptr< VectorDouble > approxVals
boost::ptr_deque< UserDataOperator > & getOpDomainRhsPipeline()
Get the Op Domain Rhs Pipeline object.
PipelineManager interface.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
boost::shared_ptr< FEMethod > & getBoundaryRhsFE()
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
SmartPetscObj< TS > createTSIM(SmartPetscObj< DM > dm=nullptr)
Create TS (time) implicit solver.
Simple interface for fast problem set-up.
Deprecated interface functions.
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
boost::ptr_deque< UserDataOperator > & getOpBoundaryLhsPipeline()
Get the Op Boundary Lhs Pipeline object.
DeprecatedCoreInterface Interface
FTensor::Tensor0< FTensor::PackPtr< double *, 1 > > getFTensor0N(const FieldApproximationBase base)
Get base function as Tensor0.
MoFEM::EdgeElementForcesAndSourcesCore EdgeEle
#define CHKERR
Inline error check.
OpCalcSurfaceAverageTemperature(boost::shared_ptr< VectorDouble > &approx_vals, double &sum_temp, double &surf)
auto createDMVector(DM dm)
Get smart vector from DM.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
[Flux Rhs]
implementation of Data Operators for Forces and Sources
#define MOFEM_LOG_C(channel, severity, format,...)
void simple(double P1[], double P2[], double P3[], double c[], const int N)
MoFEM::FaceElementForcesAndSourcesCore FaceEle
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
static auto getFTensor0FromVec(ublas::vector< T, A > &data)
Get tensor rank 0 (scalar) form data vector.
Get value at integration points for scalar field.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
boost::shared_ptr< MatrixDouble > approxGradVals
MoFEMErrorCode iNtegrate(EntData &row_data, EntData &col_data)
[Radiation Lhs]
Modify integration weights on face to take in account higher-order geometry.
OpRadiationLhs(boost::shared_ptr< VectorDouble > &approx_vals)
MoFEMErrorCode setBoundaryLhsIntegrationRule(RuleHookFun rule)
MoFEMErrorCode setDomainRhsIntegrationRule(RuleHookFun rule)
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
boost::ptr_deque< UserDataOperator > & getOpDomainLhsPipeline()
Get the Op Domain Lhs Pipeline object.
VectorShallowArrayAdaptor< double > VectorAdaptor
friend class UserDataOperator
FTensor::Index< 'i', SPACE_DIM > i
MoFEMErrorCode iNtegrate(EntData &row_data)
[Radiation Lhs]
FormsIntegrators< DomainEleOp >::Assembly< A >::LinearForm< I >::OpGradTimesTensor< 1, FIELD_DIM, SPACE_DIM > OpGradTimesTensor
constexpr double heat_conductivity
default operator for EDGE element
MoFEMErrorCode setDomainLhsIntegrationRule(RuleHookFun rule)
constexpr double emissivity
int nbCols
number if dof on column
boost::shared_ptr< FEMethod > & getDomainLhsFE()
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
int nbRows
number of dofs on rows
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpGradGrad< 1, 1, SPACE_DIM > OpDomainGradGrad
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode bC()
[Create common data]
boost::shared_ptr< VectorDouble > approxVals
MoFEMErrorCode setupProblem()
[Run problem]
ForcesAndSourcesCore::UserDataOperator UserDataOperator
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpGradGrad< 1, 1, 2 > OpDomainGradGrad
constexpr double T_ambient
boost::ptr_deque< UserDataOperator > & getOpBoundaryRhsPipeline()
Get the Op Boundary Rhs Pipeline object.
MoFEMErrorCode runProblem()
[Run problem]
MoFEMErrorCode setBoundaryRhsIntegrationRule(RuleHookFun rule)
constexpr double boltzmann_constant
int main(int argc, char *argv[])
[Check results]
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode createCommonData()
[Set up problem]
ElementsAndOps< SPACE_DIM >::DomainEle DomainEle
MoFEMErrorCode postProcess()
[Integrate]
MoFEMErrorCode iNtegrate(EntData &row_data)
[Radiation Lhs]
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
boost::shared_ptr< VectorDouble > approxVals
EntitiesFieldData::EntData EntData
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
OpRadiationRhs(boost::shared_ptr< VectorDouble > &approx_vals)
Post post-proc data at points from hash maps.