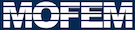 |
| v0.14.0
|
Go to the documentation of this file.
18 using namespace MoFEM;
20 static char help[] =
"...\n\n";
52 if (
auto e_ptr = field_ents[0]) {
54 entsIndices.push_back(boost::make_shared<MyStorage>(data.
getIndices()));
56 e_ptr->getWeakStoragePtr() = entsIndices.back();
58 MOFEM_LOG(
"SYNC", Sev::inform) <<
"Set " << e_ptr->getEntTypeName() <<
" "
59 << side <<
" : " << entsIndices.size();
64 if (
auto cast_ptr = boost::dynamic_pointer_cast<MyStorage>(ptr))
65 MOFEM_LOG(
"SYNC", Sev::noisy) <<
"Cast works";
71 "Pointer to stored data is expected to be set");
74 if (
auto ptr = e_ptr->getSharedStoragePtr<
MyStorage>()) {
76 << data.
getIndices() <<
" : " << ptr->globalIndices;
79 "Pointer to stored data is expected to be set");
84 "Pointer to entity should be set by finite element");
91 static std::vector<SharedVecInt>
112 if (
auto e_ptr = field_ents[0]) {
115 <<
"Test " << e_ptr->getEntTypeName() <<
" " << side;
119 if (
auto ptr = e_ptr->getSharedStoragePtr<
MyStorage>()) {
122 << data.
getIndices() <<
" : " << ptr->globalIndices;
126 auto diff = data.
getIndices() - ptr->globalIndices;
127 auto dot = inner_prod(diff, diff);
133 "Pointer to stored data is expected to be set");
138 "Pointer to entity should be set by finite element");
153 std::vector<double>
v(nb_dofs, 1);
167 int main(
int argc,
char *argv[]) {
183 DMType dm_name =
"DMMOFEM";
203 auto dm = simple_interface->
getDM();
205 auto domain_fe = boost::make_shared<VolEle>(m_field);
207 domain_fe->getRuleHook =
VolRule();
211 CHKERR m_field.
get_moab().get_entities_by_dimension(0, 3, ents,
true);
214 CHKERR skin.find_skin(0, ents,
false, skin_ents);
216 ParallelComm *pcomm =
219 CHKERR pcomm->filter_pstatus(skin_ents,
220 PSTATUS_SHARED | PSTATUS_MULTISHARED,
221 PSTATUS_NOT, -1, &proc_skin);
223 for (
auto d : {0, 1, 2})
225 moab::Interface::UNION);
230 auto get_mark_skin_dofs = [&](
Range &&skin) {
232 auto marker_ptr = boost::make_shared<std::vector<unsigned char>>();
241 auto mark_dofs = get_mark_skin_dofs(
get_skin());
244 domain_fe->getOpPtrVector().push_back(
new OpVolumeSet(
"FIELD"));
245 domain_fe->getOpPtrVector().push_back(
new OpVolumeTest(
"FIELD"));
247 domain_fe->getOpPtrVector().push_back(
248 new OpSetBc(
"FIELD",
true, mark_dofs));
250 domain_fe->getOpPtrVector().push_back(
new OpUnSetBc(
"FIELD"));
260 CHKERR VecGhostUpdateBegin(
v, ADD_VALUES, SCATTER_REVERSE);
261 CHKERR VecGhostUpdateEnd(
v, ADD_VALUES, SCATTER_REVERSE);
262 CHKERR VecGhostUpdateBegin(
v, INSERT_VALUES, SCATTER_FORWARD);
263 CHKERR VecGhostUpdateEnd(
v, INSERT_VALUES, SCATTER_FORWARD);
266 INSERT_VALUES, SCATTER_REVERSE);
276 auto loc_idx = dof->getPetscLocalDofIdx();
278 if (dof->getFieldData() != array[loc_idx])
280 "Data inconsistency");
282 if ((*mark_dofs)[loc_idx]) {
283 if (array[loc_idx] != 0)
285 "Dof assembled, but it should be not");
287 if (array[loc_idx] == 0)
289 "Dof not assembled, but it should be");
293 CHKERR VecRestoreArray(
v, &array);
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Data on single entity (This is passed as argument to DataOperator::doWork)
const VectorFieldEntities & getFieldEntities() const
get field entities
#define MYPCOMM_INDEX
default communicator number PCOMM
#define MOFEM_LOG_CHANNEL(channel)
Set and reset channel.
Problem manager is used to build and partition problems.
boost::shared_ptr< MyStorage > SharedVecInt
virtual MPI_Comm & get_comm() const =0
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
Operator set cache stored data, in this example, global indices, but it can be any structure.
Set integration rule to volume elements.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Test if cached data can be accessed, and check consistency of data.
Simple interface for fast problem set-up.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
Deprecated interface functions.
DeprecatedCoreInterface Interface
PetscErrorCode DMCreateGlobalVector_MoFEM(DM dm, Vec *g)
DMShellSetCreateGlobalVector.
MoFEMErrorCode getOptions()
get options
MyStorage(VectorInt &data)
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
OpVolumeAssemble(const std::string &field_name, Vec v)
friend class UserDataOperator
virtual moab::Interface & get_moab()=0
implementation of Data Operators for Forces and Sources
default operator for TRI element
OpVolumeSet(const std::string &field_name)
#define MOFEM_LOG_SYNCHRONISE(comm)
Synchronise "SYNC" channel.
friend class UserDataOperator
const VectorInt & getIndices() const
Get global indices of dofs on entity.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
auto & getNumeredRowDofsPtr() const
get access to numeredRowDofsPtr storing DOFs on rows
const std::string getDomainFEName() const
Get the Domain FE Name.
MoFEMErrorCode markDofs(const std::string problem_name, RowColData rc, const enum MarkOP op, const Range ents, std::vector< unsigned char > &marker) const
Create vector with marked indices.
static auto get_skin(MoFEM::Interface &m_field, Range body_ents)
int operator()(int, int, int) const
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
Volume finite element base.
constexpr auto field_name
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
int main(int argc, char *argv[])
const double v
phase velocity of light in medium (cm/ns)
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
UBlasVector< int > VectorInt
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
Set indices on entities on finite element.
OpVolumeTest(const std::string &field_name)
const FTensor::Tensor2< T, Dim, Dim > Vec
@ MOFEM_DATA_INCONSISTENCY
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
static std::vector< SharedVecInt > entsIndices
This is global static storage.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
@ MOFEM_ATOM_TEST_INVALID
keeps basic data about problem
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
const std::string getProblemName() const
Get the Problem Name.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode addDomainField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...