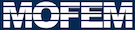 |
| v0.14.0
|
- Examples
- heat_equation.cpp.
Definition at line 91 of file heat_equation.cpp.
◆ HeatEquation()
◆ assembleSystem()
- Examples
- heat_equation.cpp.
Definition at line 205 of file heat_equation.cpp.
208 auto add_domain_lhs_ops = [&](
auto &pipeline) {
212 "U",
"U", [](
double,
double,
double) ->
double {
return k; }));
216 return c * fe_domain_lhs->ts_a;
222 auto add_domain_rhs_ops = [&](
auto &pipeline) {
225 auto grad_u_at_gauss_pts = boost::make_shared<MatrixDouble>();
226 auto dot_u_at_gauss_pts = boost::make_shared<VectorDouble>();
228 "U", grad_u_at_gauss_pts));
232 "U", grad_u_at_gauss_pts,
233 [](
double,
double,
double) ->
double {
return k; }));
235 "U", dot_u_at_gauss_pts,
236 [](
const double,
const double,
const double) {
return c; }));
237 auto source_term = [&](
const double x,
const double y,
const double z) {
240 const auto t = fe_domain_lhs->ts_t;
241 return 1e1 * pow(M_E, -M_PI * M_PI *
t) * sin(1. * M_PI * x) *
248 auto add_boundary_lhs_ops = [&](
auto &pipeline) {
251 "U",
"U", [](
const double,
const double,
const double) {
return c; }));
254 auto add_boundary_rhs_ops = [&](
auto &pipeline) {
256 auto u_at_gauss_pts = boost::make_shared<VectorDouble>();
257 auto boundary_function = [&](
const double x,
const double y,
261 const auto t = fe_rhs->ts_t;
269 [](
const double,
const double,
const double) {
return c; }));
275 add_domain_lhs_ops(pipeline_mng->getOpDomainLhsPipeline());
276 add_domain_rhs_ops(pipeline_mng->getOpDomainRhsPipeline());
277 add_boundary_lhs_ops(pipeline_mng->getOpBoundaryLhsPipeline());
278 add_boundary_rhs_ops(pipeline_mng->getOpBoundaryRhsPipeline());
◆ boundaryCondition()
- Examples
- heat_equation.cpp.
Definition at line 183 of file heat_equation.cpp.
188 CHKERR bc_mng->pushMarkDOFsOnEntities(
simple->getProblemName(),
"ESSENTIAL",
191 auto &bc_map = bc_mng->getBcMapByBlockName();
192 boundaryMarker = boost::make_shared<std::vector<char unsigned>>();
193 for (
auto b : bc_map) {
194 if (std::regex_match(b.first, std::regex(
"(.*)ESSENTIAL(.*)"))) {
196 for (
int i = 0;
i != b.second->bcMarkers.size(); ++
i) {
197 (*boundaryMarker)[
i] |= b.second->bcMarkers[
i];
◆ initialCondition()
◆ outputResults()
◆ readMesh()
◆ runProgram()
◆ setIntegrationRules()
◆ setupProblem()
◆ solveSystem()
That to work, you have to create solver, as follows,
That is explicitly use use Simple DM to create solver for DM. Pipeline menage by default creat copy of DM, in case several solvers are used the same DM.
Alternatively you can get dm directly from the solver, i.e.
DM ts_dm;
CHKERR TSGetDM(solver, &ts_dm);
- Examples
- heat_equation.cpp.
Definition at line 300 of file heat_equation.cpp.
306 auto create_post_process_element = [&]() {
307 auto post_proc_fe = boost::make_shared<PostProcEle>(
mField);
308 auto det_ptr = boost::make_shared<VectorDouble>();
309 auto jac_ptr = boost::make_shared<MatrixDouble>();
310 auto inv_jac_ptr = boost::make_shared<MatrixDouble>();
312 post_proc_fe->getOpPtrVector().push_back(
314 post_proc_fe->getOpPtrVector().push_back(
317 auto u_ptr = boost::make_shared<VectorDouble>();
318 post_proc_fe->getOpPtrVector().push_back(
323 post_proc_fe->getOpPtrVector().push_back(
325 new OpPPMap(post_proc_fe->getPostProcMesh(),
326 post_proc_fe->getMapGaussPts(),
339 auto set_time_monitor = [&](
auto dm,
auto solver) {
341 boost::shared_ptr<Monitor> monitor_ptr(
342 new Monitor(dm, create_post_process_element()));
343 boost::shared_ptr<ForcesAndSourcesCore>
null;
345 monitor_ptr,
null,
null);
349 auto set_fieldsplit_preconditioner = [&](
auto solver) {
352 CHKERR TSGetSNES(solver, &snes);
354 CHKERR SNESGetKSP(snes, &ksp);
356 CHKERR KSPGetPC(ksp, &pc);
357 PetscBool is_pcfs = PETSC_FALSE;
358 PetscObjectTypeCompare((PetscObject)pc, PCFIELDSPLIT, &is_pcfs);
360 if (is_pcfs == PETSC_TRUE) {
362 auto name_prb =
simple->getProblemName();
363 auto is_all_bc = bc_mng->getBlockIS(name_prb,
"ESSENTIAL",
"U", 0, 0);
365 CHKERR ISGetSize(is_all_bc, &is_all_bc_size);
367 <<
"Field split block size " << is_all_bc_size;
368 CHKERR PCFieldSplitSetIS(pc, PETSC_NULL,
391 auto set_user_ts_jacobian = [&](
auto dm) {
397 auto dm =
simple->getDM();
401 auto solver = pipeline_mng->createTSIM(
404 CHKERR set_user_ts_jacobian(dm);
405 CHKERR set_time_monitor(dm, solver);
406 CHKERR TSSetSolution(solver,
D);
407 CHKERR TSSetFromOptions(solver);
408 CHKERR set_fieldsplit_preconditioner(solver);
◆ boundaryMarker
boost::shared_ptr<std::vector<unsigned char> > HeatEquation::boundaryMarker |
|
private |
◆ mField
The documentation for this struct was generated from the following file:
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpBaseTimesScalar< 1 > OpBoundaryTimeScalarField
boost::shared_ptr< FEMethod > & getDomainRhsFE()
static PetscErrorCode set(TS ts, PetscReal t, Vec u, Vec u_t, PetscReal a, Mat A, Mat B, void *ctx)
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpBaseTimesScalar< 1 > OpDomainTimesScalarField
OpCalculateScalarFieldValuesFromPetscVecImpl< PetscData::CTX_SET_X_T > OpCalculateScalarFieldValuesDot
PipelineManager interface.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
boost::shared_ptr< FEMethod > & getBoundaryRhsFE()
Simple interface for fast problem set-up.
MoFEMErrorCode initialCondition()
MoFEMErrorCode setupProblem()
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, 1 > OpDomainSource
Get field gradients at integration pts for scalar filed rank 0, i.e. vector field.
MoFEMErrorCode boundaryCondition()
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, 1 > OpBoundaryMass
#define CHKERR
Inline error check.
auto createDMVector(DM dm)
Get smart vector from DM.
virtual moab::Interface & get_moab()=0
Simple interface for fast problem set-up.
void simple(double P1[], double P2[], double P3[], double c[], const int N)
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
Get value at integration points for scalar field.
MoFEMErrorCode setIntegrationRules()
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpGradGrad< 1, 1, SPACE_DIM > OpDomainGradGrad
MoFEM::Interface & mField
constexpr double t
plate stiffness
FTensor::Index< 'i', SPACE_DIM > i
Add operators pushing bases from local to physical configuration.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpGradTimesTensor< 1, 1, SPACE_DIM > OpDomainGradTimesVec
boost::shared_ptr< FEMethod > & getDomainLhsFE()
MoFEMErrorCode outputResults()
#define MOFEM_LOG(channel, severity)
Log.
MoFEMErrorCode solveSystem()
[Push operators to pipeline]
PetscErrorCode DMMoFEMTSSetMonitor(DM dm, TS ts, const std::string fe_name, boost::shared_ptr< MoFEM::FEMethod > method, boost::shared_ptr< MoFEM::BasicMethod > pre_only, boost::shared_ptr< MoFEM::BasicMethod > post_only)
Set Monitor To TS solver.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
Set indices on entities on finite element.
Interface for managing meshsets containing materials and boundary conditions.
boost::shared_ptr< std::vector< unsigned char > > boundaryMarker
const double D
diffusivity
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, 1 > OpBoundarySource
MoFEMErrorCode readMesh()
bool checkMeshset(const int ms_id, const CubitBCType cubit_bc_type) const
check for CUBIT Id and CUBIT type
MoFEMErrorCode assembleSystem()
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
auto getDMTsCtx(DM dm)
Get TS context data structure used by DM.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, 1 > OpDomainMass
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
Post post-proc data at points from hash maps.